getCookies
Get browser cookies for the current domain or the specified domain.
Syntax​
cy.getCookies()
cy.getCookies(options)
Usage​
Correct Usage
cy.getCookies() // Get cookies for the currrent domain
Arguments​
options (Object)
Pass in an options object to change the default behavior of cy.getCookies()
.
Option | Default | Description |
---|---|---|
domain | Hostname of the current URL | Retrieves the cookies from the specified domain |
log | true | Displays the command in the Command log |
timeout | responseTimeout | Time to wait for cy.getCookies() to resolve before timing out |
Yields ​
cy.getCookies()
yields an array of cookie objects. Each cookie object has the
following properties:
domain
: (String)expiry
: (Number) (if specified)hostOnly
: (Boolean) (if specified)httpOnly
: (Boolean)name
: (String)path
: (String)sameSite
: (String) (if specified)secure
: (Boolean)value
: (String)
cy.getCookies()
is not a query. It will not update the returned list if
further cookies are added after it initially executes.
Examples​
Get Cookies​
Get cookies after logging in​
In this example, on first login our server sends us back a session cookie.
// assume we just logged in
cy.contains('Login').click()
cy.url().should('include', 'profile')
cy.getCookies()
.should('have.length', 1)
.then((cookies) => {
expect(cookies[0]).to.have.property('name', 'session_id')
})
Rules​
Requirements ​
cy.getCookies()
requires being chained off ofcy
.
Assertions ​
cy.getCookies()
will only run assertions you have chained once, and will not retry.
Timeouts ​
cy.getCookies()
should never time out.
Because cy.getCookies()
is asynchronous it is technically possible for there
to be a timeout while talking to the internal Cypress automation APIs. But for
practical purposes it should never happen.
Command Log​
cy.getCookies()
.should('have.length', 1)
.then((cookies) => {
expect(cookies[0]).to.have.property('name', 'fakeCookie1')
expect(cookies[0]).to.have.property('value', '123ABC')
expect(cookies[0]).to.have.property('domain')
expect(cookies[0]).to.have.property('httpOnly')
expect(cookies[0]).to.have.property('path')
expect(cookies[0]).to.have.property('secure')
})
The commands above will display in the Command Log as:
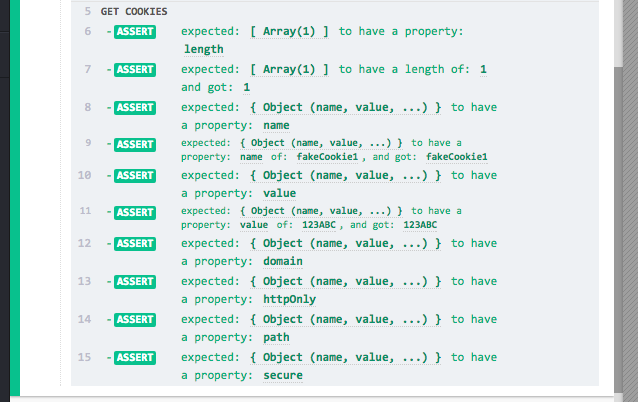
When clicking on getCookies
within the command log, the console outputs the
following:
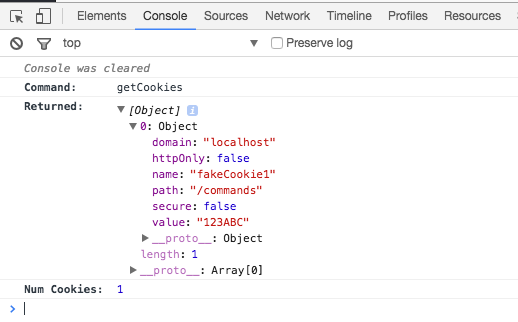
History​
Version | Changes |
---|---|
5.0.0 | Removed experimentalGetCookiesSameSite and made sameSite property always available. |
4.3.0 | Added sameSite property when the experimentalGetCookiesSameSite configuration value is true . |