getCookie
Get a browser cookie by its name.
Syntax​
cy.getCookie(name)
cy.getCookie(name, options)
Usage​
Correct Usage
cy.getCookie('auth_key') // Get cookie with name 'auth_key'
Arguments​
name (String)
The name of the cookie to get. Required.
options (Object)
Pass in an options object to change the default behavior of cy.getCookie()
.
Option | Default | Description |
---|---|---|
domain | Hostname of the current URL | Retrieves the cookie from the specified domain |
log | true | Displays the command in the Command log |
timeout | responseTimeout | Time to wait for cy.getCookie() to resolve before timing out |
Yields ​
cy.getCookie()
yields a cookie object with the following properties:
domain
expiry
(if specified)hostOnly
(if specified)httpOnly
name
path
sameSite
(if specified)secure
value
cy.getCookie()
is not a query. It will not retry, or wait for the requested
cookie to exist.
When a cookie matching the name could not be found:​
cy.getCookie()
yields null
.
Examples​
Session id​
Get session_id
cookie after logging in​
In this example, on first login, our server sends us back a session cookie.
// assume we just logged in
cy.contains('Login').click()
cy.url().should('include', 'profile')
// retries until cookie with value=189jd09su
// is found or default command timeout ends
cy.getCookie('session_id')
.should('have.property', 'value', '189jd09su')
.then((cookie) => {
// cookie is an object with "domain", "name" and other properties
})
You can check the cookie existence without comparing any of its properties
cy.getCookie('my-session-cookie').should('exist')
If you need the cookie value, for example to use in a subsequent call
let cookie
cy.getCookie('session_id')
.should('exist')
.then((c) => {
// save cookie until we need it
cookie = c
})
// some time later, force the "cy.request"
// to run ONLY after the cookie has been set
// by placing it inside ".then"
cy.get('#submit')
.click()
.then(() => {
cy.request({
url: '/api/admin',
headers: {
'my-token-x': cookie.value,
},
})
})
Using cy.getCookie()
to test logging in​
Check out our example recipes using cy.getCookie()
to test
logging in using HTML web forms,
logging in using XHR web forms and
logging in with single sign on
Rules​
Requirements ​
cy.getCookie()
requires being chained off ofcy
.
Assertions ​
cy.getCookie()
will only run assertions you have chained once, and will not retry.
Timeouts ​
cy.getCookie()
should never time out.
Because cy.getCookie()
is asynchronous it is technically possible for there to
be a timeout while talking to the internal Cypress automation APIs. But for
practical purposes it should never happen.
Command Log​
Get a browser cookie and make assertions about the object
cy.getCookie('fakeCookie1').should('have.property', 'value', '123ABC')
The commands above will display in the Command Log as:

When clicking on getCookie
within the command log, the console outputs the
following:
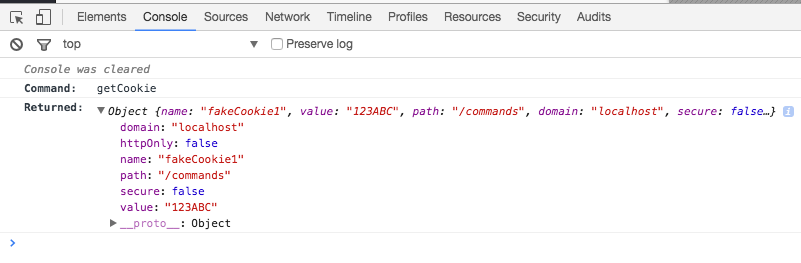
History​
Version | Changes |
---|---|
5.0.0 | Removed experimentalGetCookiesSameSite and made sameSite property always available. |
4.3.0 | Added sameSite property when the experimentalGetCookiesSameSite configuration value is true . |