get
Get one or more DOM elements by selector or alias.
The querying behavior of this command is similar to how
$(...)
works in jQuery.
Syntax​
cy.get(selector)
cy.get(alias)
cy.get(selector, options)
cy.get(alias, options)
Usage​
Correct Usage
cy.get('.list > li') // Yield the <li>'s in .list
Arguments​
selector (String selector)
A selector used to filter matching DOM elements.
alias (String)
An alias as defined using the .as()
command and referenced
with the @
character and the name of the alias.
You can use cy.get()
for aliases of primitives, regular objects, or even DOM
elements.
When using aliases with DOM elements, Cypress will query the DOM again if the previously aliased DOM element has gone stale.
options (Object)
Pass in an options object to change the default behavior of cy.get()
.
Option | Default | Description |
---|---|---|
log | true | Displays the command in the Command log |
timeout | defaultCommandTimeout | Time to wait for cy.get() to resolve before timing out |
withinSubject | null | Element to search for children in. If null, search begins from root-level DOM element |
includeShadowDom | includeShadowDom config option value | Whether to traverse shadow DOM boundaries and include elements within the shadow DOM in the yielded results. |
Yields ​
cy.get()
yields the DOM element(s) it found, or the results of the alias lookup.cy.get()
is a query, and it is safe to chain further commands.
Examples​
Selector​
Get the input element​
cy.get('input').should('be.disabled')
Find the first li
descendent within a ul
​
cy.get('ul li:first').should('have.class', 'active')
Find the dropdown-menu and click it​
cy.get('.dropdown-menu').click()
Find 5 elements with the given data attribute​
cy.get('[data-test-id="test-example"]').should('have.length', 5)
Find the link with an href attribute containing the word "questions" and click it​
cy.get('a[href*="questions"]').click()
Find the element with id that starts with "local-"​
cy.get('[id^=local-]')
Find the element with id that ends with "-remote"​
cy.get('[id$=-remote]')
Find the element with id that starts with "local-" and ends with "-remote"​
cy.get('[id^=local-][id$=-remote]')
Find the element with id that has characters used in CSS like ".", ":".​
cy.get('#id\\.\\.\\.1234') // escape the character with \\
cy.get()
in the .within()
command​
Since cy.get()
is chained off of cy
, it always looks for the selector within
the entire document
. The only exception is when used inside a
.within() command.
cy.get('form').within(() => {
cy.get('input').type('Pamela') // Only yield inputs within form
cy.get('textarea').type('is a developer') // Only yield textareas within form
})
Get vs Find​
The cy.get
command always starts its search from the
cy.root element. In most cases, it is the
document
element, unless used inside the .within()
command. The .find command starts its search from
the current subject.
<div class="test-title">cy.get vs .find</div>
<section id="comparison">
<div class="feature">Both are querying commands</div>
</section>
cy.get('#comparison')
.get('div')
// finds the div.test-title outside the #comparison
// and the div.feature inside
.should('have.class', 'test-title')
.and('have.class', 'feature')
cy.get('#comparison')
.find('div')
// the search is limited to the tree at #comparison element
// so it finds div.feature only
.should('have.length', 1)
.and('have.class', 'feature')
Alias​
For a detailed explanation of aliasing, read more about aliasing here.
Get the aliased 'todos' elements​
cy.get('ul#todos').as('todos')
//...hack hack hack...
//later retrieve the todos
cy.get('@todos')
Get the aliased 'submitBtn' element​
beforeEach(() => {
cy.get('button[type=submit]').as('submitBtn')
})
it('disables on click', () => {
cy.get('@submitBtn').should('be.disabled')
})
Get the aliased 'users' fixture​
beforeEach(() => {
cy.fixture('users.json').as('users')
})
it('disables on click', () => {
// access the array of users
cy.get('@users').then((users) => {
// get the first user
const user = users[0]
cy.get('header').contains(user.name)
})
})
Rules​
Requirements ​
cy.get()
requires being chained off a command that yields DOM element(s).
Assertions ​
cy.get()
will automatically retry until the element(s) exist in the DOM.cy.get()
will automatically retry until all chained assertions have passed.
Timeouts ​
cy.get()
can time out waiting for the element(s) to exist in the DOM.cy.get()
can time out waiting for assertions you've added to pass.
Command Log​
Get an input and assert on the value
cy.get('input[name="firstName"]').should('have.value', 'Homer')
The commands above will display in the Command Log as:

When clicking on the get
command within the command log, the console outputs
the following:
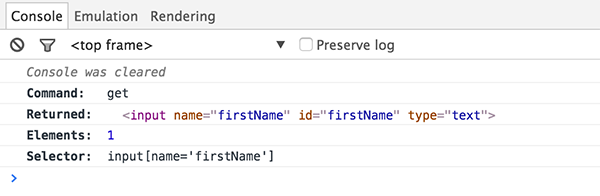
History​
Version | Changes |
---|---|
5.2.0 | Added includeShadowDom option. |