eq
Get A DOM element at a specific index in an array of elements.
info
The querying behavior of this command matches exactly how
.eq()
works in jQuery.
Syntax​
.eq(index)
.eq(indexFromEnd)
.eq(index, options)
.eq(indexFromEnd, options)
Usage​
Correct Usage
cy.get('tbody>tr').eq(0) // Yield first 'tr' in 'tbody'
cy.get('ul>li').eq(4) // Yield fifth 'li' in 'ul'
Incorrect Usage
cy.eq(0) // Errors, cannot be chained off 'cy'
cy.getCookies().eq(4) // Errors, 'getCookies' does not yield DOM element
Arguments​
index (Number)
A number indicating the index to find the element at within an array of elements. Starts with 0.
indexFromEnd (Number)
A negative number indicating the index position from the end to find the element at within an array of elements.
options (Object)
Pass in an options object to change the default behavior of .eq()
.
Option | Default | Description |
---|---|---|
log | true | Displays the command in the Command log |
timeout | defaultCommandTimeout | Time to wait for .eq() to resolve before timing out |
Yields ​
.eq()
yields the new DOM element it found..eq()
is a query, and it is safe to chain further commands.
Examples​
Index​
Find the 2nd element within the elements​
<ul>
<li>tabby</li>
<li>siamese</li>
<li>persian</li>
<li>sphynx</li>
<li>burmese</li>
</ul>
cy.get('li').eq(1).should('contain', 'siamese') // true
Make an assertion on the 3rd row of a table​
<table>
<tr>
<th>Breed</th>
<th>Origin</th>
</tr>
<tr>
<td>Siamese</td>
<td>Thailand</td>
</tr>
<tr>
<td>Sphynx</td>
<td>Canada</td>
</tr>
<tr>
<td>Persian</td>
<td>Iran</td>
</tr>
</table>
cy.get('tr').eq(2).should('contain', 'Canada') //true
Index From End​
Find the 2nd from the last element within the elements​
<ul>
<li>tabby</li>
<li>siamese</li>
<li>persian</li>
<li>sphynx</li>
<li>burmese</li>
</ul>
cy.get('li').eq(-2).should('contain', 'sphynx') // true
Rules​
Requirements ​
.eq()
requires being chained off a command that yields DOM element(s).
Assertions ​
.eq()
will automatically retry until the element(s) exist in the DOM..eq()
will automatically retry until all chained assertions have passed.
Timeouts ​
.eq()
can time out waiting for the element(s) to exist in the DOM..eq()
can time out waiting for assertions you've added to pass.
Command Log​
Find the 4th <li>
in the navigation
cy.get('.left-nav.nav').find('>li').eq(3)
The commands above will display in the Command Log as:

When clicking on the eq
command within the command log, the console outputs
the following:
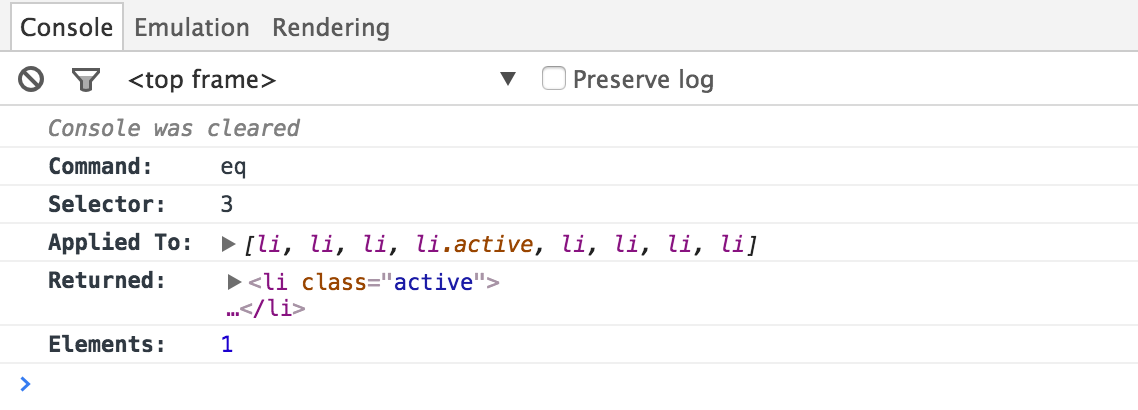