select
Select an <option>
within a <select>
.
It is unsafe to
chain further commands that rely on the subject after .select()
.
Syntax​
.select(value)
.select(values)
.select(value, options)
.select(values, options)
Usage​
Correct Usage
cy.get('select').select('user-1') // Select the 'user-1' option
Incorrect Usage
cy.select('John Adams') // Errors, cannot be chained off 'cy'
cy.clock().select() // Errors, 'clock' does not yield a <select> element
Arguments​
value (String, Number)
The value
, index
, or text content of the <option>
to be selected.
values (Array)
An array of values
, indexes
, or text contents of the <option>
s to be
selected.
options (Object)
Pass in an options object to change the default behavior of .select()
.
Option | Default | Description |
---|---|---|
force | false | Forces the action, disables waiting for actionability |
log | true | Displays the command in the Command log |
timeout | defaultCommandTimeout | Time to wait for .select() to resolve before timing out |
Yields ​
.select()
yields the same subject it was given.- It is unsafe
to chain further commands that rely on the subject after
.select()
.
Examples​
Text Content​
Select the option
with the text apples
​
<select>
<option value="456">apples</option>
<option value="457">oranges</option>
<option value="458">bananas</option>
</select>
// yields <option value="456">apples</option>
cy.get('select').select('apples').should('have.value', '456')
Value​
Select the option
with the value "456"​
<select>
<option value="456">apples</option>
<option value="457">oranges</option>
<option value="458">bananas</option>
</select>
// yields <option value="456">apples</option>
cy.get('select').select('456').should('have.value', '456')
Index​
Select the option
with index 0​
<select>
<option value="456">apples</option>
<option value="457">oranges</option>
<option value="458">bananas</option>
</select>
// yields <option value="456">apples</option>
cy.get('select').select(0).should('have.value', '456')
Select multiple options​
Select the options with the texts "apples" and "bananas"​
<select multiple>
<option value="456">apples</option>
<option value="457">oranges</option>
<option value="458">bananas</option>
</select>
cy.get('select')
.select(['apples', 'bananas'])
.invoke('val')
.should('deep.equal', ['456', '458'])
Select the options with the values "456" and "457"​
<select multiple>
<option value="456">apples</option>
<option value="457">oranges</option>
<option value="458">bananas</option>
</select>
cy.get('select')
.select(['456', '457'])
.invoke('val')
.should('deep.equal', ['456', '457'])
Select the options with the indexes 0 and 1​
<select multiple>
<option value="456">apples</option>
<option value="457">oranges</option>
<option value="458">bananas</option>
</select>
cy.get('select')
.select([0, 1])
.invoke('val')
.should('deep.equal', ['456', '457'])
Note: Passing an array into cy.select()
will select only the options
matching values in the array, leaving all other options unselected (even those
that were previously selected). In the same manner, calling cy.select([])
with
an empty array will clear selections on all options.
Force select​
Force select a hidden <select>
​
<select style="display: none;">
<optgroup label="Fruits">
<option value="banana">Banana</option>
<option value="apple">Apple</option>
</optgroup>
<optgroup></optgroup>
</select>
cy.get('select')
.select('banana', { force: true })
.invoke('val')
.should('eq', 'banana')
Force select a disabled <select>
​
Passing { force: true }
to .select()
will override the actionability checks
for selecting a disabled <select>
. However, it will not override the
actionability checks for selecting a disabled <option>
or an option within a
disabled <optgroup>
. See
this issue for more detail.
<select disabled>
<optgroup label="Veggies">
<option value="okra">Okra</option>
<option value="zucchini">Zucchini</option>
</optgroup>
<optgroup></optgroup>
</select>
cy.get('select')
.select('okra', { force: true })
.invoke('val')
.should('eq', 'okra')
Selected option​
You can get the currently selected option using the jQuery's :selected selector.
<select id="name">
<option>Joe</option>
<option>Mary</option>
<option selected="selected">Peter</option>
</select>
cy.get('select#name option:selected').should('have.text', 'Peter')
Notes​
Actionability​
.select()
is an action command that follows the rules of
Actionability.
However, passing { force: true }
to .select()
will not override the
actionability checks for selecting a disabled <option>
or an option within a
disabled <optgroup>
. See
this issue for more detail.
Rules​
Requirements ​
.select()
requires being chained off a command that yields DOM element(s)..select()
requires the element to be aselect
.
Assertions ​
.select()
will automatically wait for the element to reach an actionable state..select()
will automatically retry until all chained assertions have passed.
Timeouts ​
.select()
can time out waiting for the element to reach an actionable state..select()
can time out waiting for assertions you've added to pass.
Command Log​
Select the option with the text "Homer Simpson"
cy.get('select').select('Homer Simpson')
The commands above will display in the Command Log as:

When clicking on select
within the command log, the console outputs the
following:
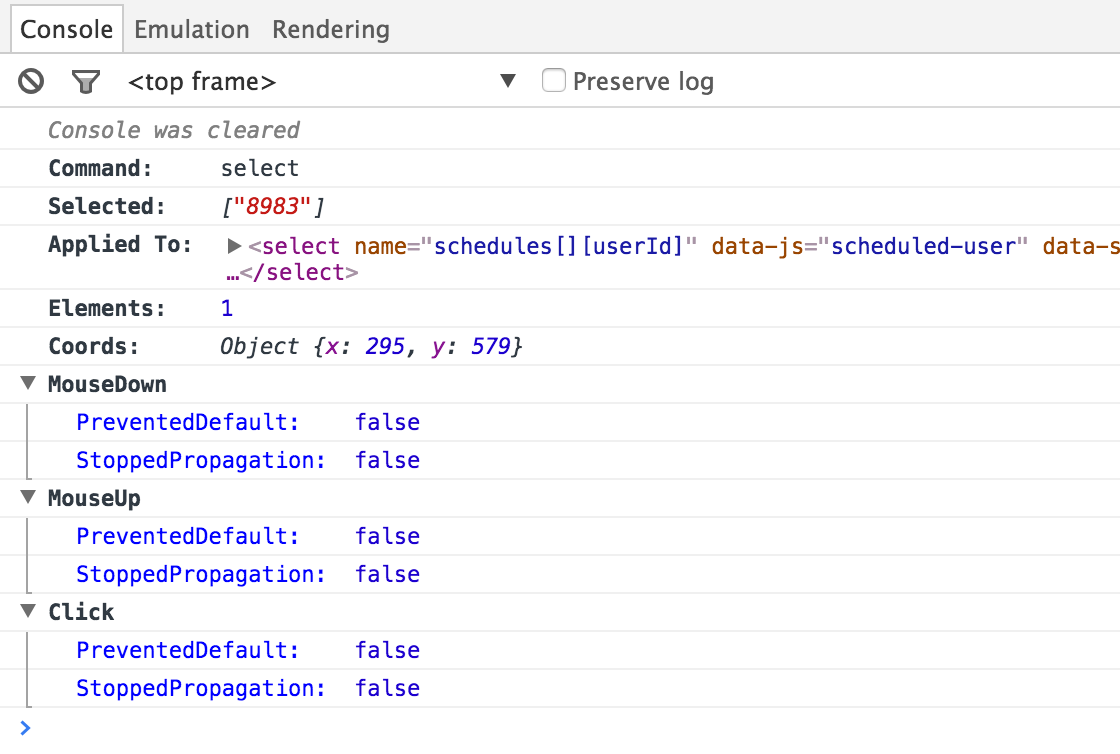