its
Get a property's value on the previously yielded subject.
info
If you want to call a function
on the previously yielded subject, use
.invoke()
.
Syntax​
.its(propertyName)
.its(propertyName, options)
Usage​
Correct Usage
cy.wrap({ width: '50' }).its('width') // Get the 'width' property
cy.window().its('sessionStorage') // Get the 'sessionStorage' property
Incorrect Usage
cy.its('window') // Errors, cannot be chained off 'cy'
cy.clearCookies().its('length') // Errors, 'clearCookies' does not yield Object
Arguments​
propertyName (String, Number)
Index, name of property or name of nested properties (with dot notation) to get.
options (Object)
Pass in an options object to change the default behavior of .its()
.
Option | Default | Description |
---|---|---|
log | true | Displays the command in the Command log |
timeout | defaultCommandTimeout | Time to wait for .its() to resolve before timing out |
Yields ​
.its()
'yields the value of the property..its()
is a query, and it is safe to chain further commands.
Examples​
Objects​
Get property​
cy.wrap({ age: 52 }).its('age').should('eq', 52) // true
Arrays​
Get index​
cy.wrap(['Wai Yan', 'Yu']).its(1).should('eq', 'Yu') // true
DOM Elements​
Get the length
property of a DOM element​
cy.get('ul li') // this yields us a jquery object
.its('length') // calls 'length' property returning that value
.should('be.gt', 2) // ensure the length is greater than 2
Requests​
Get the user
object of the response's body
​
cy
.request(...)
.its('body.user')
.then(user => ...)
alternatively, use destructuring
cy
.request(...)
.its('body')
.then(({user}) => ...)
Strings​
Get length
of title​
cy.title().its('length').should('eq', 24)
Functions​
Get function as property​
const fn = () => {
return 42
}
cy.wrap({ getNum: fn }).its('getNum').should('be.a', 'function')
Access function properties​
You can access functions to then drill into their own properties instead of invoking them.
// Your app code
// a basic Factory constructor
const Factory = (arg) => {
// ...
}
Factory.create = (arg) => {
return new Factory(arg)
}
// assign it to the window
window.Factory = Factory
cy.window() // yields window object
.its('Factory') // yields Factory function
.invoke('create', 'arg') // now invoke properties on it
Use .its()
to test window.fetch
​
Nested Properties​
You can drill into nested properties by using dot notation.
const user = {
contacts: {
work: {
name: 'Kamil',
},
},
}
cy.wrap(user).its('contacts.work.name').should('eq', 'Kamil') // true
Existence​
Wait for some property to exist on window
​
cy.window()
.its('globalProp')
.then((globalProp) => {
// do something now that window.globalProp exists
})
Assert that a property does not exist on window
​
cy.window().its('evilProp').should('not.exist')
Rules​
Requirements ​
.its()
requires being chained off a previous command.
Assertions ​
.its()
will automatically retry until it has a property that is notnull
orundefined
.
Timeouts ​
.its()
can time out waiting for the property to exist..its()
can time out waiting for assertions you've added to pass.
Command Log​
Get responseBody
of aliased route
cy.intercept('/comments', { fixture: 'comments.json' }).as('getComments')
cy.get('#fetch-comments').click()
cy.wait('@getComments')
.its('response.body')
.should(
'deep.eq',
JSON.stringify([
{ id: 1, comment: 'hi' },
{ id: 2, comment: 'there' },
])
)
The commands above will display in the Command Log as:
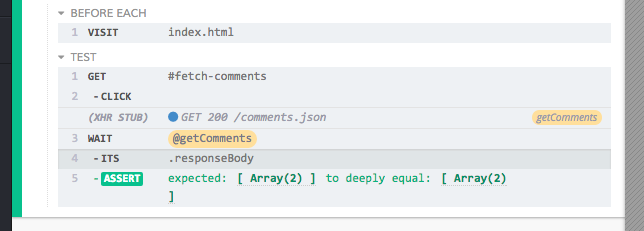
When clicking on its
within the command log, the console outputs the
following:
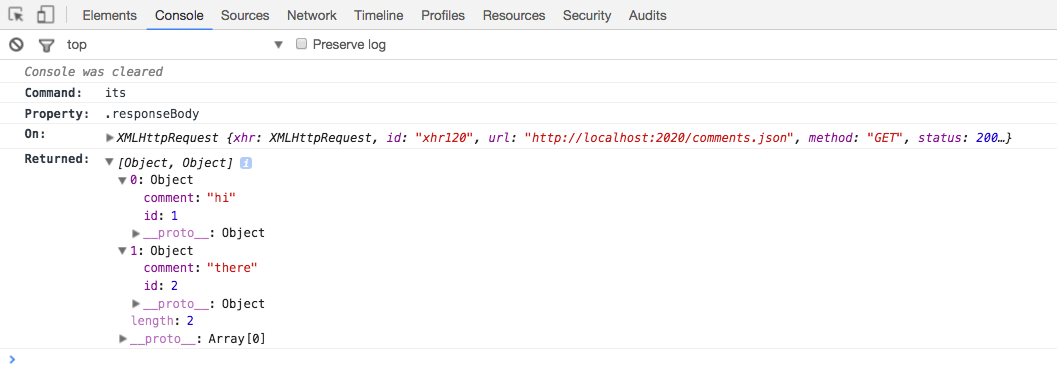
History​
Version | Changes |
---|---|
3.8.0 | Added support for options argument |
3.7.0 | Added support for arguments of type Number for propertyName |