click
Click a DOM element.
It is unsafe to
chain further commands that rely on the subject after .click()
.
Syntax​
.click()
.click(options)
.click(position)
.click(position, options)
.click(x, y)
.click(x, y, options)
Usage​
Correct Usage
cy.get('.btn').click() // Click on button
cy.focused().click() // Click on el with focus
cy.contains('Welcome').click() // Click on first el containing 'Welcome'
Incorrect Usage
cy.click('.btn') // Errors, cannot be chained off 'cy'
cy.window().click() // Errors, 'window' does not yield DOM element
Arguments​
position (String)
The position where the click should be issued. The center
position is the
default position. Valid positions are topLeft
, top
, topRight
, left
,
center
, right
, bottomLeft
, bottom
, and bottomRight
.
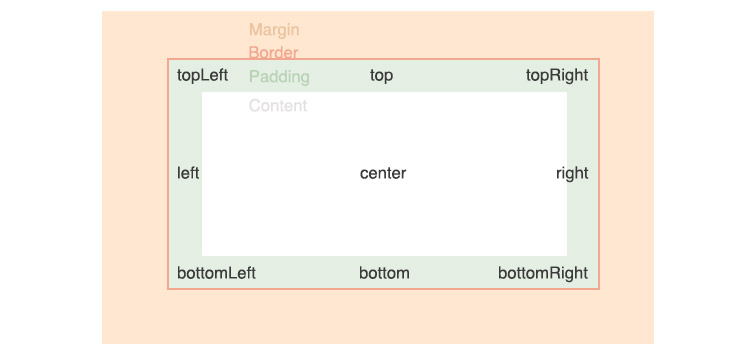
x (Number)
The distance in pixels from the element's left to issue the click.
y (Number)
The distance in pixels from the element's top to issue the click.
options (Object)
Pass in an options object to change the default behavior of .click()
.
Option | Default | Description |
---|---|---|
altKey | false | Activates the alt key (option key for Mac). Aliases: optionKey . |
animationDistanceThreshold | animationDistanceThreshold | The distance in pixels an element must exceed over time to be considered animating. |
ctrlKey | false | Activates the control key. Aliases: controlKey . |
log | true | Displays the command in the Command log |
force | false | Forces the action, disables waiting for actionability |
metaKey | false | Activates the meta key (Windows key or command key for Mac). Aliases: commandKey , cmdKey . |
multiple | false | Serially click multiple elements |
scrollBehavior | scrollBehavior | Viewport position to where an element should be scrolled before executing the command |
shiftKey | false | Activates the shift key. |
timeout | defaultCommandTimeout | Time to wait for .click() to resolve before timing out |
waitForAnimations | waitForAnimations | Whether to wait for elements to finish animating before executing the command. |
Yields ​
.click()
yields the same subject it was given.- It is unsafe
to chain further commands that rely on the subject after
.click()
.
Examples​
No Args​
Click a link in a nav​
cy.get('.nav > a').click()
Position​
Specify a corner of the element to click​
Click the top right corner of the button.
cy.get('img').click('topRight')
Coordinates​
Specify explicit coordinates relative to the top left corner​
The click below will be issued inside of the element (15px from the left and 40px from the top).
cy.get('#top-banner').click(15, 40)
Options​
Force a click regardless of its actionable state​
Forcing a click overrides the actionable checks Cypress applies and will automatically fire the events.
cy.get('.close').as('closeBtn')
cy.get('@closeBtn').click({ force: true })
Force a click with position argument​
cy.get('#collapse-sidebar').click('bottomLeft', { force: true })
Force a click with relative coordinates​
cy.get('#footer .next').click(5, 60, { force: true })
Click all elements with id starting with 'btn'​
By default, Cypress will error if you're trying to click multiple elements. By
passing { multiple: true }
Cypress will iteratively apply the click to each
element and will also log to the
Command Log multiple times.
cy.get('[id^=btn]').click({ multiple: true })
Click with key combinations​
The .click()
command may also be fired with key modifiers in order to simulate
holding key combinations while clicking, such as ALT + click
.
You can also use key combinations during .type(). This offers options to hold down keys across multiple commands. See Key Combinations for more information.
The following keys can be combined with .click()
through the options
.
Option | Notes |
---|---|
altKey | Activates the alt key (option key for Mac). Aliases: optionKey . |
ctrlKey | Activates the control key. Aliases: controlKey . |
metaKey | Activates the meta key (Windows key or command key for Mac). Aliases: commandKey , cmdKey . |
shiftKey | Activates the shift key. |
Shift click​
// execute a SHIFT + click on the first <li>
cy.get('li:first').click({
shiftKey: true,
})
Notes​
Actionability​
The element must first reach actionability​
.click()
is an "action command" that follows all the rules of
Actionability.
Focus​
Focus is given to the first focusable element​
For example, clicking a <span>
inside of a <button>
gives the focus to the
button, since that's what would happen in a real user scenario.
However, Cypress additionally handles situations where a child descendent is clicked inside of a focusable parent, but actually isn't visually inside the parent (per the CSS Object Model). In those cases if no focusable parent is found the window is given focus instead (which matches real browser behavior).
Cancellation​
Mousedown cancellation will not cause focus​
If the mousedown event has its default action prevented ( e.preventDefault()
)
then the element will not receive focus as per the spec.
Rules​
Requirements ​
.click()
requires being chained off a command that yields DOM element(s).
Assertions ​
.click()
will automatically wait for the element to reach an actionable state..click()
will automatically retry until all chained assertions have passed.
Timeouts ​
.click()
can time out waiting for the element to reach an actionable state..click()
can time out waiting for assertions you've added to pass.
Command Log​
Click the button
cy.get('.action-btn').click()
The commands above will display in the Command Log as:

When clicking on click
within the command log, the console outputs the
following:
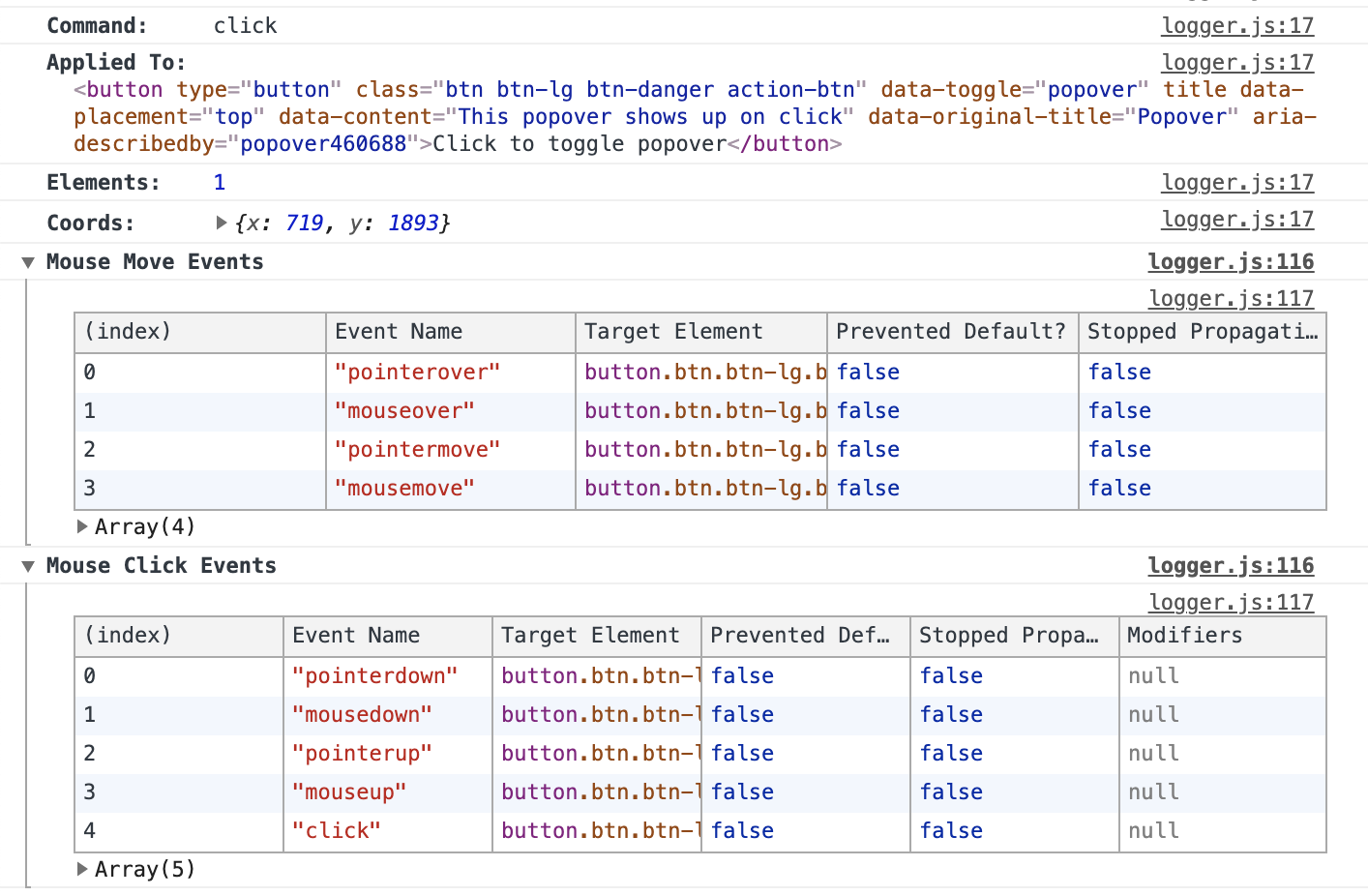
History​
Version | Changes |
---|---|
6.1.0 | Added option scrollBehavior |
3.5.0 | Added sending mouseover , mousemove , mouseout , pointerdown , pointerup , and pointermove during .click() |