as
Assign an alias for later use. Reference the alias later within a
cy.get()
query or cy.wait()
command with an @
prefix.
Note: .as()
assumes you are already familiar with core concepts such as
aliases
Syntax​
.as(aliasName)
.as(aliasName, options)
Usage​
Correct Usage
cy.get('.main-nav').find('li').first().as('firstNav') // Alias element as @firstNav
cy.get('input.username').invoke('val').as('username', { type: 'static' }) // Alias that references the value at the time the alias was created
cy.intercept('PUT', '/users').as('putUser') // Alias route as @putUser
cy.stub(api, 'onUnauth').as('unauth') // Alias stub as @unauth
cy.spy(win, 'fetch').as('winFetch') // Alias spy as @winFetch
Incorrect Usage
cy.as('foo') // Errors, cannot be chained off 'cy'
Arguments​
aliasName (String)
The name of the alias to be referenced later within a
cy.get()
or cy.wait()
command
using an @
prefix.
options (Object)
Pass in an options object to change the default behavior of .as()
.
Option | Default | Description |
---|---|---|
type | query | The type of alias to store, which impacts how the value is retrieved later in the test. Valid values are query and static . A query alias re-runs all queries leading up to the resulting value each time the alias is requested. A static alias is retrieved once when the alias is stored, and will never change. type has no effect when aliasing intercepts, spies, and stubs. |
Yields ​
.as()
yields the same subject it was given.- It is safe to chain further commands after
.as()
.
Examples​
DOM element​
Aliasing a DOM element and then using cy.get()
to access
the aliased element.
it('disables on click', () => {
cy.get('button[type=submit]').as('submitBtn')
cy.get('@submitBtn').click().should('be.disabled')
})
Intercept​
Aliasing an intercepted route defined with
cy.intercept()
and then using
cy.wait()
to wait for the aliased route.
// `PUT` requests on the `/users` endpoint will be stubbed with
// the `user` fixture and be aliased as `editUser`
cy.intercept('PUT', '/users', { fixture: 'user' }).as('editUser')
// we'll assume submitting `form` triggers a matching request
cy.get('form').submit()
// once a response comes back from the `editUser`
// this `wait` will resolve with the subject containing `url`
cy.wait('@editUser').its('url').should('contain', 'users')
More examples of aliasing routes can be found here.
Fixture​
Aliasing cy.fixture()
data and then using this
to
access it via the alias.
beforeEach(() => {
cy.fixture('users-admins.json').as('admins')
})
it('the users fixture is bound to this.admins', function () {
cy.log(`There are ${this.admins.length} administrators.`)
})
Note the use of the standard function syntax. Using
arrow functions
to access aliases via this
won't work because of
the lexical binding
of this
.
Notes​
Aliases are reset​
Note: all aliases are reset before each test. See the aliases guide for details.
Reserved words​
Alias names cannot match some reserved words.​
Some strings are not allowed as alias names since they are reserved words in
Cypress. These words include: test
, runnable
, timeout
, slow
, skip
, and
inspect
.
as
is asynchronous​
Remember that Cypress commands are async, including .as()
.
Because of this you cannot synchronously access anything you have aliased. You
must use other asynchronous commands such as .then()
to
access what you've aliased.
Here are some further examples of using .as()
that illustrate the asynchronous
behavior.
describe('A fixture', () => {
describe('alias can be accessed', () => {
it('via get().', () => {
cy.fixture('admin-users.json').as('admins')
cy.get('@admins').then((users) => {
cy.log(`There are ${users.length} admins.`)
})
})
it('via then().', function () {
cy.fixture('admin-users.json').as('admins')
cy.visit('/').then(() => {
cy.log(`There are ${this.admins.length} admins.`)
})
})
})
describe('aliased in beforeEach()', () => {
beforeEach(() => {
cy.fixture('admin-users.json').as('admins')
})
it('is bound to this.', function () {
cy.log(`There are ${this.admins.length} admins.`)
})
})
})
Rules​
Requirements ​
.as()
requires being chained off a previous command.
Assertions ​
.as()
is a utility command..as()
will not run assertions. Assertions will pass through as if this command did not exist.
Timeouts ​
.as()
cannot time out.
Command Log​
Alias several routes
cy.intercept('/company/*').as('companyGet')
cy.intercept('/roles/*').as('rolesGet')
cy.intercept('/teams/*').as('teamsGet')
cy.intercept(/users\/\d+/).as('userGet')
cy.intercept('PUT', /^\/users\/\d+/).as('userPut')
Aliases of routes display in the routes instrument panel:
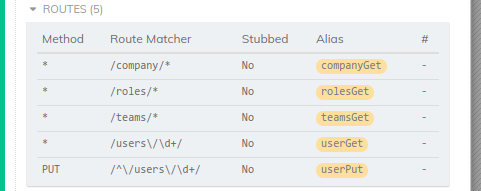
History​
Version | Changes |
---|---|
12.3.0 | Added option type to opt into the pre-12.0.0 behavior. |
12.0.0 | All aliases now re-run queries leading up to them by default. |