Cypress Configuration
What you'll learn
- How to configure Cypress to change the default behavior of Cypress
- Configuration options available in Cypress
- How to view and override configuration at runtime
This guide is for Cypress 10+.
For more info on upgrading configuration to Cypress 10, see the migration guide.
Configuration File
Launching Cypress for the first time, you will be guided through a wizard that
will create a Cypress configuration file for you. This file will be
cypress.config.js
for JavaScript apps or cypress.config.ts
for
TypeScript apps. This file is used to
store any configuration specific to Cypress.
Cypress additionally supports config files with .mjs
or .cjs
extensions.
Using a .mjs
file will allow you to use
ESM Module
syntax in your config without the need of a transpiler step.
A '.cjs' file uses the CommonJS module syntax, which is the default for JavaScript files. All JavaScript config examples in our docs use the CommonJS format.
If you configure your tests to record the
results to Cypress Cloud the
projectId
will be stored in the config file as well.
Intelligent Code Completion
The defineConfig
helper function is exported by Cypress, and it provides
automatic code completion for configuration in many popular code editors. While
it's not strictly necessary for Cypress to parse your configuration, we
recommend wrapping your config object with defineConfig()
like this:
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
e2e: {
baseUrl: 'http://localhost:1234',
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
e2e: {
baseUrl: 'http://localhost:1234',
},
})
Options
The default behavior of Cypress can be modified by supplying any of the following configuration options. Below is a list of available options and their default values.
Global
Option | Default | Description |
---|---|---|
clientCertificates | [] | An optional array of client certificates. |
env | {} | Any values to be set as environment variables. |
includeShadowDom | false | Whether to traverse shadow DOM boundaries and include elements within the shadow DOM in the results of query commands (e.g. cy.get() ). |
numTestsKeptInMemory | Default based on run or open mode | The number of tests for which snapshots and command data are kept in memory. numTestsKeptInMemory is set to 50 by default during cypress open and set to 0 by default during cypress run . Reduce this number if you are experiencing high memory consumption in your browser during a test run. |
port | null | Port used to host Cypress. Normally this is a randomly generated port. |
redirectionLimit | 20 | The number of times that the application under test can redirect before erroring. |
reporter | spec | The reporter used during cypress run . |
reporterOptions | null | The reporter options used. Supported options depend on the reporter. |
retries | { "runMode": 0, "openMode": 0 } | The number of times to retry a failing test. Can be configured to apply to cypress run or cypress open separately. As of Cypress 13.4.0 , the experimental Flake Detection strategy can also be configured. See Test Retries for more information. |
watchForFileChanges | Default based on run or open mode | Whether Cypress will watch and restart tests on test file changes. watchForFileChanges is set to true by default during cypress open and set to false by default during cypress run . |
Timeouts
Timeouts are a core concept you should understand well. The default values listed here are meaningful.
Option | Default | Description |
---|---|---|
defaultCommandTimeout | 4000 | Time, in milliseconds, to wait until most DOM based commands are considered timed out. |
execTimeout | 60000 | Time, in milliseconds, to wait for a system command to finish executing during a cy.exec() command. |
taskTimeout | 60000 | Time, in milliseconds, to wait for a task to finish executing during a cy.task() command. |
pageLoadTimeout | 60000 | Time, in milliseconds, to wait for page transition events or cy.visit() , cy.go() , cy.reload() commands to fire their page load events. Network requests are limited by the underlying operating system, and may still time out if this value is increased. |
requestTimeout | 5000 | Time, in milliseconds, to wait for a request to go out in a cy.wait() command. |
responseTimeout | 30000 | Time, in milliseconds, to wait until a response in a cy.request() , cy.wait() , cy.fixture() , cy.getCookie() , cy.getCookies() , cy.setCookie() , cy.clearCookie() , cy.clearCookies() , and cy.screenshot() commands. |
Folders / Files
Option | Default | Description |
---|---|---|
downloadsFolder | cypress/downloads | Path to folder where files downloaded during a test are saved. |
fileServerFolder | root project folder | Path to folder where application files will attempt to be served from. |
fixturesFolder | cypress/fixtures | Path to folder containing fixture files (Pass false to disable). |
screenshotsFolder | cypress/screenshots | Path to folder where screenshots will be saved from cy.screenshot() command or after a test fails during cypress run . |
videosFolder | cypress/videos | Path to folder where videos will be saved during cypress run . |
Screenshots
Option | Default | Description |
---|---|---|
screenshotOnRunFailure | true | Whether Cypress will take a screenshot when a test fails during cypress run . |
screenshotsFolder | cypress/screenshots | Path to folder where screenshots will be saved from cy.screenshot() command or after a test fails during cypress run . |
trashAssetsBeforeRuns | true | Whether Cypress will trash assets within the downloadsFolder , screenshotsFolder , and videosFolder before tests run with cypress run . |
For more options regarding screenshots, view the Cypress.Screenshot API.
Videos
Currently, Cypress supports video recording for supported Chromium-based browsers (Chrome/Electron/Edge). Support for Firefox can be tracked in this issue.
Option | Default | Description |
---|---|---|
trashAssetsBeforeRuns | true | Whether Cypress will trash assets within the downloadsFolder , screenshotsFolder , and videosFolder before tests run with cypress run . |
videoCompression | false | The quality setting for the video compression, in Constant Rate Factor (CRF). The value can be false or 0 to disable compression or a CRF between 1 and 51 , where a lower value results in better quality (at the expense of a higher file size). Setting this option to true will result in a default CRF of 32 . |
videosFolder | cypress/videos | Where Cypress will automatically save the video of the test run when tests run with cypress run . |
video | false | Whether Cypress will capture a video of the tests run with cypress run . |
Downloads
Option | Default | Description |
---|---|---|
downloadsFolder | cypress/downloads | Path to folder where files downloaded during a test are saved. |
trashAssetsBeforeRuns | true | Whether Cypress will trash assets within the downloadsFolder , screenshotsFolder , and videosFolder before tests run with cypress run . |
Browser
Option | Default | Description |
---|---|---|
defaultBrowser | null | The default browser to launch if the "--browser" command line option is not provided. This option only affects the first browser launch; changing this option after the browser is already launched will have no effect. |
chromeWebSecurity | true | Whether to enable Chromium-based browser's Web Security for same-origin policy and insecure mixed content. |
blockHosts | null | A String or Array of hosts that you wish to block traffic for. Please read the notes for examples on using this. |
modifyObstructiveCode | true | Whether Cypress will search for and replace obstructive JS code in .js or .html files. Please read the notes for more information on this setting. |
userAgent | null | Enables you to override the default user agent the browser sends in all request headers. User agent values are typically used by servers to help identify the operating system, browser, and browser version. See User-Agent MDN Documentation for example user agent values. |
Viewport
Option | Default | Description |
---|---|---|
viewportHeight | 660 | Default height in pixels for the application under tests' viewport. (Override with cy.viewport() command) |
viewportWidth | 1000 | Default width in pixels for the application under tests' viewport. (Override with cy.viewport() command) |
Actionability
Option | Default | Description |
---|---|---|
animationDistanceThreshold | 5 | The distance in pixels an element must exceed over time to be considered animating. |
waitForAnimations | true | Whether to wait for elements to finish animating before executing commands. |
scrollBehavior | top | Viewport position to which an element should be scrolled before executing commands. Can be 'center' , 'top' , 'bottom' , 'nearest' , or false . false disables scrolling. |
For more information, see the docs on actionability.
Experiments
Configuration might include experimental options currently being tested. See Experiments page.
Testing Type-Specific Options
You can provide configuration options for either E2E or Component Testing by
creating e2e
and component
objects inside your Cypress configuration.
e2e
These options are available to be specified inside the e2e
configuration
object:
Option | Default | Description |
---|---|---|
baseUrl | null | URL used as prefix for cy.visit() or cy.request() command's URL. |
setupNodeEvents | null | Function in which node events can be registered and config can be modified. Takes the place of the (removed) pluginFile option. Please read the notes for examples on using this. |
supportFile | cypress/support/e2e.{js,jsx,ts,tsx} | Path to file to load before spec files load. This file is compiled and bundled. (Pass false to disable) |
specPattern | cypress/e2e/**/*.cy.{js,jsx,ts,tsx} | A String or Array of glob patterns of the test files to load. |
excludeSpecPattern | *.hot-update.js | A String or Array of glob patterns used to ignore test files that would otherwise be shown in your list of tests. Please read the notes on using this. |
experimentalRunAllSpecs | false | Enables the "Run All Specs" UI feature, allowing the execution of multiple specs sequentially. |
slowTestThreshold | 10000 | Time, in milliseconds, to consider a test "slow" during cypress run . A slow test will display in orange text in the default reporter. |
testIsolation | true | Whether or not test isolation is enabled to ensure a clean browser context between tests. |
injectDocumentDomain deprecated | false | Instructs Cypress to use the document.domain property to reduce the need for cy.origin . Please read the notes on using this. |
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
e2e: {
// e2e options here
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
e2e: {
// e2e options here
},
})
component
These options are available to be specified inside the component
configuration
object:
Option | Default | Description |
---|---|---|
devServer | null | Required option used to configure the component testing dev server. Please read the notes for examples on using this. |
indexHtmlFile | cypress/support/component-index.html | This is where Cypress renders your components and allows you to add in global assets, such as styles, fonts, and external scripts. |
justInTimeCompile | true | Compile resources directly related to your spec for webpack, compiling them 'just-in-time' before spec execution. |
setupNodeEvents | null | Function in which node events can be registered and config can be modified. Takes the place of the (removed) plugins file. Please read the notes for examples on using this. |
supportFile | cypress/support/component.js | Path to file to load before spec files load. This file is compiled and bundled. (Pass false to disable) |
specPattern | **/*.cy.{js,jsx,ts,tsx} | A glob pattern String or Array of glob pattern Strings of the spec files to load. Note that any files found matching the e2e.specPattern value will be automatically excluded. |
excludeSpecPattern | ['/snapshots/*', '/image_snapshots/*'] | A String or Array of glob patterns used to ignore spec files that would otherwise be shown in your list of specs. Please read the notes on using this. |
experimentalSingleTabRunMode | false | Run all specs in a single tab, instead of creating a new tab per spec. This can improve run mode performance, but can impact spec isolation and reliability on large test suites. This experiment currently only applies to Component Testing. |
slowTestThreshold | 250 | Time, in milliseconds, to consider a test "slow" during cypress run . A slow test will display in orange text in the default reporter. |
devServerPublicPathRoute | /__cypress/src | The public path of the dev server in use. Use caution overriding the default value as it can have unintended consequences. See Framework Configuration or dev server specific documentation (in particular @cypress/vite-dev-server ). |
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
component: {
// component options here
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
component: {
// component options here
},
})
Overriding Options
Cypress gives you the option to dynamically alter configuration options. This is helpful when running Cypress in multiple environments and on multiple developer machines.
Overriding Individual Options
When running Cypress from the command line you can pass a --config
flag to
override individual config options.
For example, to override viewportWidth
and viewportHeight
, you can run:
cypress run --browser firefox --config viewportWidth=1280,viewportHeight=720
Specifying an Alternative Config File
In the Cypress CLI, you can change which config file Cypress will use with the
--config-file
flag.
cypress run --config-file tests/cypress.config.js
See the Command Line guide for more examples.
Testing Type-Specific Overrides
In addition to setting Testing Type-Specific options, you can override other configuration options for either the E2E Testing or Component Testing.
For example:
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
// These settings apply everywhere unless overridden
defaultCommandTimeout: 5000,
viewportWidth: 1000,
viewportHeight: 600,
// Viewport settings overridden for component tests
component: {
viewportWidth: 500,
viewportHeight: 500,
},
// Command timeout overridden for E2E tests
e2e: {
defaultCommandTimeout: 10000,
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
// These settings apply everywhere unless overridden
defaultCommandTimeout: 5000,
viewportWidth: 1000,
viewportHeight: 600,
// Viewport settings overridden for component tests
component: {
viewportWidth: 500,
viewportHeight: 500,
},
// Command timeout overridden for E2E tests
e2e: {
defaultCommandTimeout: 10000,
},
})
Environment Variables
Configuration options can be overridden with environment variables. This is especially useful in Continuous Integration or when working locally. This gives you the ability to change configuration options without modifying any code or build scripts.
For example, these environment variables in the command line will override any
viewportWidth
or viewportHeight
options set in the Cypress configuration:
export CYPRESS_VIEWPORT_WIDTH=800
export CYPRESS_VIEWPORT_HEIGHT=600
See the Environment Variables guide for more examples.
Test Configuration
We provide two options to override the configuration while your test are
running, Cypress.config()
and suite-specific or test-specific configuration
overrides.
⚠️ Note: The configuration values below are all writeable and can be changed via per test configuration. Any other configuration values are readonly and cannot be changed at run time.
animationDistanceThreshold
baseUrl
blockHosts
defaultCommandTimeout
env
note: Provided environment variables will be merged with current environment variables.execTimeout
includeShadowDom
keystrokeDelay
numTestsKeptInMemory
pageLoadTimeout
redirectionLimit
requestTimeout
responseTimeout
retries
screenshotOnRunFailure
scrollBehavior
slowTestThreshold
taskTimeout
testIsolation
- this option can only be overridden at the suite-specific override levelviewportHeight
viewportWidth
waitForAnimations
Cypress.config()
You can also override configuration values within your test using
Cypress.config()
.
This changes the configuration for the remaining execution of the current spec file. The values will reset to the previous default values after the spec has complete.
Cypress.config('pageLoadTimeout', 100000)
Cypress.config('pageLoadTimeout') // => 100000
Test-specific Configuration
To apply specific Cypress configuration values to a suite or test, pass a configuration object to the test or suite function as the second argument.
The configuration values passed in will only take effect during the suite or test where they are set. The values will then reset to the previous default values after the suite or test is complete.
Syntax
describe(name, config, fn)
context(name, config, fn)
it(name, config, fn)
specify(name, config, fn)
Suite configuration
If you want to target a suite of tests to run or be excluded when run in a
specific browser, you can override the browser
configuration within the suite
configuration. The browser
option accepts the same arguments as
Cypress.isBrowser()
.
You can configure the number of times to retries a suite of tests if they fail
during cypress run
and cypress open
separately.
describe(
'login',
{
retries: {
runMode: 3,
openMode: 2,
},
},
() => {
it('should redirect unauthenticated user to sign-in page', () => {
// ...
})
it('allows user to login', () => {
// ...
})
}
)
Single test configuration
If you want to target a test to run or be excluded when run in a specific
browser, you can override the browser
configuration within the test
configuration. The browser
option accepts the same arguments as
Cypress.isBrowser().
it('Show warning outside Chrome', { browser: '!chrome' }, () => {
cy.get('.browser-warning').should(
'contain',
'For optimal viewing, use Chrome browser'
)
})
Resolved Configuration
When you open a Cypress project, expanding the Project Settings panel under Settings will display the resolved configuration to you. This helps you to understand and see where different values came from. Each set value is highlighted to show where the value has been set via the following ways:
- Default value
- Cypress configuration file
- The Cypress environment file
- System environment variables
- Command Line arguments
- setupNodeEvents
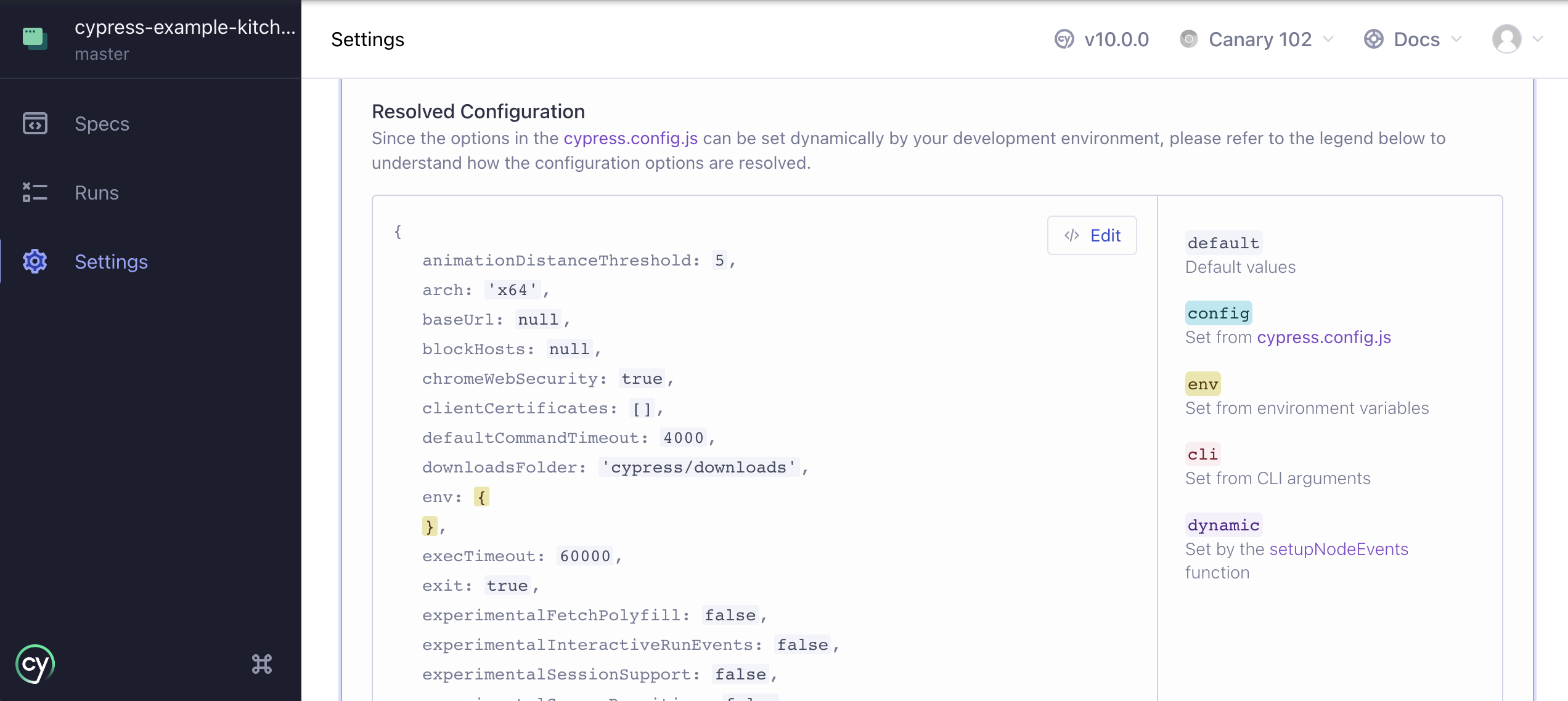
Notes
blockHosts
By passing a string or array of strings you can block requests made to one or more hosts.
To block a host:
- ✅ Pass only the host
- ✅ Use wildcard
*
patterns - ✅ Include the port other than
80
and443
- ❌ Do NOT include protocol:
http://
orhttps://
Not sure what a part of the URL a host is? Use this guide as a reference.
When blocking a host, we use minimatch
to check
the host. When in doubt you can test whether something matches yourself.
Given the following URLs:
https://www.google-analytics.com/ga.js
http://localhost:1234/some/user.json
This would match the following blocked hosts:
www.google-analytics.com
*.google-analytics.com
*google-analytics.com
localhost:1234
Because localhost:1234
uses a port other than 80
and 443
it must be
included.
Be cautious for URL's which have no subdomain.
For instance given a URL: https://google.com/search?q=cypress
- ✅ Matches
google.com
- ✅ Matches
*google.com
- ❌ Does NOT match
*.google.com
When Cypress blocks a request made to a matching host, it will automatically
send a 503
status code. As a convenience it also sets a
x-cypress-matched-blocked-host
header so you can see which rule it
matched.
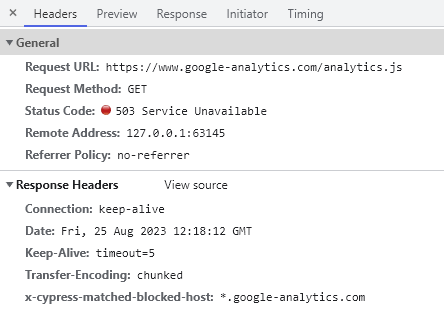
devServer
The devServer
option is required for component
testing, and
allows you to register a component testing dev server.
Typically, you will specify a framework
and bundler
options in devServer
for your framework and UI library like so:
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
component: {
devServer: {
framework: 'create-react-app',
bundler: 'webpack',
},
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
component: {
devServer: {
framework: 'create-react-app',
bundler: 'webpack',
},
},
})
See
Framework Configuration
guide for more info on all the available framework
and bundler
options, as
well as additional configuration options.
Custom Dev Server
It is possible to customize the devServer and provide your own function for custom or advanced setups.
The devServer function receives a cypressConfig
argument:
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
component: {
devServer(cypressConfig) {
// return dev server instance or a promise that resolves to
// a dev server instance here
},
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
component: {
devServer(cypressConfig) {
// return dev server instance or a promise that resolves to
// a dev server instance here
},
},
})
See the Custom Dev Server guide for more info.
excludeSpecPattern
Cypress uses minimatch
with the options: {dot: true, matchBase: true}
. We
suggest using https://globster.xyz to test what files
would match.
The **/node_modules/**
pattern is automatically added to excludeSpecPattern
,
and does not need to be specified (and can't be overridden). See e2e
or component
testing-specific options.
injectDocumentDomain
This option is deprecated, and will be removed in a future version of Cypress.
Set this configuration option to true
to instruct Cypress to
inject document.domain
into your test application.
This can reduce the need for cy.origin()
when navigating between subdomains,
but comes with compatibility caveats for some sites.
This configuration option is provided to ease the transition between cy.origin()
's behavior
in Cypress 13 and the default behavior in Cypress 14.
Read the Cypress 14 migration guide to understand how to update your tests to remove
the need to set this flag.
This configuration value must be set to true if you are running tests with experimental
WebKit support, as cy.origin()
is not yet supported in WebKit.
Known Incompatibilities
Setting this configuration value to true
can cause the application you are testing
to behave in unexpected ways. This is especially true if a site your tests visit
sets the Origin-Agent-Cluster
header.
At this point in time, we are aware of the following sites that cannot be tested properly
if this option is set to true
:
- Azure AD B2C authentication workflows
- Salesforce
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
e2e: {
injectDocumentDomain: true,
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
e2e: {
injectDocumentDomain: true,
},
})
isInteractive
You can open Cypress in the interactive mode via the cypress open
command, and
in run mode via the cypress run
command. To detect the mode from your test
code you can query the isInteractive
property on
Cypress.config.
if (Cypress.config('isInteractive')) {
// interactive "cypress open" mode!
} else {
// "cypress run" mode
}
modifyObstructiveCode
With this option enabled - Cypress will search through the response streams
coming from your server on .html
and .js
files and replace code that matches
patterns commonly found in framebusting.
These script patterns are antiquated and deprecated security techniques to prevent clickjacking and framebusting. They are a relic of the past and are no longer necessary in modern browsers. However many sites and applications still implement them.
These techniques prevent Cypress from working, and they can be safely removed without altering any of your application's behavior.
Cypress modifies these scripts at the network level, and therefore there is a tiny performance cost to search the response streams for these patterns.
You can turn this option off if the application or site you're testing does not implement these security measures. Additionally it's possible that the patterns we search for may accidentally rewrite valid JS code. If that's the case, please disable this option.
Details for experimentalModifyObstructiveThirdPartyCode
can be found
here.
setupNodeEvents
The setupNodeEvents
function allows you to tap into, modify, or extend the
internal behavior of Cypress using the on
and config
arguments, and is valid as
an e2e
or component
testing specific option.
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
e2e: {
setupNodeEvents(on, config) {
// e2e testing node events setup code
},
},
component: {
setupNodeEvents(on, config) {
// component testing node events setup code
},
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
e2e: {
setupNodeEvents(on, config) {
// e2e testing node events setup code
},
},
component: {
setupNodeEvents(on, config) {
// component testing node events setup code
},
},
})
See the plugins guide for more information.
Common problems
baseUrl
is not set
Make sure you do not accidentally place the baseUrl
config option into the
env
object. The following configuration is incorrect and will not work:
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
// ⛔️ DOES NOT WORK
env: {
baseUrl: 'http://localhost:3030',
FOO: 'bar',
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
// ⛔️ DOES NOT WORK
env: {
baseUrl: 'http://localhost:3030',
FOO: 'bar',
},
})
Solution: place the baseUrl
property outside the env
object and inside the
e2e testing-type specific object.
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
// ✅ THE CORRECT WAY
env: {
FOO: 'bar',
},
e2e: {
baseUrl: 'http://localhost:3030',
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
// ✅ THE CORRECT WAY
env: {
FOO: 'bar',
},
e2e: {
baseUrl: 'http://localhost:3030',
},
})
You can also find a few tips on setting the baseUrl
in this
short video.
Test files not found when using spec
parameter
When using the --spec <path or mask>
argument, make it relative to the
project's folder. If the specs are still missing, run Cypress with
DEBUG logs with the
following setting to see how Cypress is looking for spec files:
DEBUG=cypress:cli,cypress:data-context:sources:FileDataSource,cypress:data-context:sources:ProjectDataSource
History
Version | Changes |
---|---|
14.0.0 | Added support for re-enabling superdomain navigation with the injectDocumentDomain configuration option |
13.16.0 | Added defaultBrowser option. |
13.4.0 | Added support for configuring the Experimental Flake Detection strategy via retries.experimentalStrategy and retries.experimentalOptions . |
13.0.0 | Removed nodeVersion option. |
13.0.0 | Removed videoUploadOnPasses option. |
11.0.0 | Removed e2e.experimentalSessionAndOrigin option. |
10.4.0 | Added e2e.testIsolation option. |
10.0.0 | Reworked page to support new cypress.config.js and deprecated cypress.json files. |
8.7.0 | Added slowTestThreshold option. |
8.0.0 | Added clientCertificates option and removed firefoxGcInterval configuration. |
7.0.0 | Added e2e and component options. |
7.0.0 | Added redirectionLimit option. |
6.1.0 | Added scrollBehavior option. |
5.2.0 | Added includeShadowDom option. |
5.0.0 | Added retries configuration. |
5.0.0 | Renamed blacklistHosts configuration to blockHosts . |
4.1.0 | Added screenshotOnRunFailure configuration. |
4.0.0 | Added firefoxGcInterval configuration. |
3.5.0 | Added nodeVersion configuration. |