Cypress App FAQ
General Cypress Questions​
Is Cypress free and open source?​
The Cypress App is a free, downloadable and open source (MIT license) application. This is always free to use.
Cypress Cloud is a web application that offers a variety of billing plans (including a free, open source plan) for when you want to record your test runs in CI.
Additionally, we have add-ons like UI Coverage and Cypress Accessibility which have separate pricing.
Please see our Pricing Page for more details.
What operating systems do you support?​
You can install Cypress on Mac, Linux, and Windows. For additional information, please see our System requirements.
Do you support native mobile apps?​
Cypress will never be able to run on a native mobile app, but we can test some functionality of mobile web browsers and test mobile applications that are developed in a browser, such as with the Ionic framework.
Currently you can control the viewport with the
cy.viewport()
command to test responsive, mobile
views in a website or web application. You can also mimic certain behaviors like
swiping using custom commands.
You can read about testing mobile applications with Ionic and Cypress here and see how we manage testing the mobile viewport in the Cypress Real World App.
How is this different from 'X' testing tool?​
The Cypress App is a hybrid application/framework/service all rolled into one. It takes a little bit of other testing tools, brings them together and improves on them.
In addition to the App, Cypress offers solutions like the Cypress Cloud, UI Coverage, and Accessibility to extend the value of your tests and gain insights into the health of your test suite.
You can see our evaluation of Cypress against some frameworks in our Migrating from Selenium and Migrating from Protractor guides.
Do you support X language or X framework?​
Any and all. Ruby, Node, C#, PHP - none of that matters. Cypress tests anything that runs in the context of a browser. It is back end, front end, language and framework agnostic.
You'll write your tests in JavaScript or TypeScript, but beyond that Cypress works everywhere.
Will Cypress work in my CI provider?​
Cypress works in any CI provider.
Does Cypress require me to change any of my existing code?​
No. But if you're wanting to test parts of your application that are not easily testable, you'll want to refactor those situations (as you would for any testing).
We use WebSockets; will Cypress work with that?​
Yes.
Is it possible to use cypress on .jspa
?​
Yes. Cypress works on anything rendered to a browser.
Can I use Cypress to script user-actions on an external site like gmail.com
?​
Using Cypress to test against a 3rd party application is not its intended use. It may work but will defeat the purpose of why it was created. You use Cypress while you develop your application, it helps you write your tests.
Is there code coverage?​
There is a plugin and detailed documentation on how to get end-to-end, unit and full stack code coverage.
- Read our Code Coverage guide
- Use the @cypress/code-coverage plugin
Are there driver bindings in my language?​
Cypress does not utilize WebDriver for testing, so it does not use or have any notion of driver bindings. If your language can be somehow transpiled to JavaScript, then you can configure Cypress webpack preprocessor or Cypress Browserify preprocessor to transpile your tests to JavaScript that Cypress can run.
What's the difference between a Spec, Test, and a Test Suite?​
The terms spec, test, and test suite have specific meanings. Here's how they are differentiated in Cypress:
Spec A single test file that contains a set of test cases or individual tests. Each spec file typically focuses on testing a specific feature, functionality, or aspect of the application under test. Specs define the test scenarios, interactions with the application, and assertions to verify expected behavior. Spec files are written in JavaScript or TypeScript and have a .js
or .ts
extension.
Test An individual test case within a spec file. It represents a specific scenario or behavior that needs to be tested. A test typically consists of a series of actions performed on the application being tested, such as interacting with UI elements, submitting forms, or making assertions to validate the expected outcomes. Cypress provides a rich set of built-in commands and APIs to facilitate writing tests and interacting with the application in a declarative manner.
Test Suite A collection of spec files that are grouped together. It allows you to organize your tests based on different criteria, such as functional areas, modules, or specific features. A test suite can include multiple spec files, each containing one or more tests. By grouping related tests together in a test suite, you can organize and manage your tests more effectively. Cypress provides options to run individual spec files, multiple spec files, or the entire test suite during test execution.
When should I write a End-to-End tests and when should I write Component Tests?​
For more information on how to choose a Cypress testing type, we recommend this Testing Types Guide.
When should I write a unit test and when should I write an end-to-end test?​
We believe unit tests and end-to-end tests have differences that should guide your choice.
Unit tests | End-to-end tests |
---|---|
Focus on code | Focus on the features |
Should be kept short | Can be long |
Examine the returned result of actions | Examine side effect of actions: DOM, storage, network, file system, database |
Important to developer workflow | Important to end user's workflow |
In addition to the above differences, below are a few rules of thumb to decide when to write a unit test and when to write an end-to-end test.
- If the code you are trying to test is called from other code, use a unit test.
- If the code is going be called from the external system, like a browser, use an end-to-end test.
- If a unit test requires a lot of mocking and you have to bring tools like
jsdom
,enzyme
, orsinon.js
to simulate a real world environment, you may want to rewrite it as an end-to-end test. - If an end-to-end test does not go through the browser and instead calls the code directly, you probably want to rewrite it as a unit test
Finally, unit and end-to-end tests are not that different and have common features. Good tests:
- Focus on and test only one thing.
- Are flake-free and do not fail randomly.
- Give you confidence to refactor code and add new features.
- Are able to run both locally and on a continuous integration server.
Certainly, unit and end-to-end tests are NOT in opposition to each other and are complementary tools in your toolbox.
How do I convince my company to use Cypress?​
First, be honest with yourself - is Cypress the right tool for your company and your project? We believe that the best approach is a "bottoms up" approach, where you can demonstrate how Cypress solves your company's particular needs. Implement a prototype with your project to see how it feels. Test a couple of common user stories. Identify if there are any technical blockers. Show the prototype to others before proceeding any further. If you can demonstrate the benefits of using Cypress as a developer tool for your project to other engineers, then Cypress will likely be more quickly adopted.
How can I find out about new versions of Cypress?​
We publish our releases at GitHub and npm, together with the releases we also publish a changelog with the principal changes, fixes, and updates. You can follow through these links:
How often are Cypress versions released?​
We try to release the Cypress App every two weeks.
If there is a significant bug outside of our release schedule then we release a patch as soon as possible.
What information is captured or transmitted when using the Cypress App?​
The Cypress App runs locally so no data is sent to Cypress aside from exception data, which can be disabled using the instructions here.
Can I write API tests using Cypress?​
Cypress is mainly designed to run end-to-end and component tests, but if you
need to write a few tests that call the backend API using the
cy.request()
command ... who can stop you?
it('adds a todo', () => {
cy.request({
url: '/todos',
method: 'POST',
body: {
title: 'Write REST API',
},
})
.its('body')
.should('deep.contain', {
title: 'Write REST API',
completed: false,
})
})
Take a look at our Real World App (RWA) that uses quite a few such tests to verify the backend APIs.
You can verify the responses using the built-in assertions and perform multiple calls. You can even write E2E tests that combine UI commands with API testing as needed:
it('adds todos', () => {
// drive the application through its UI
cy.visit('/')
cy.get('.new-todo')
.type('write E2E tests{enter}')
.type('add API tests as needed{enter}')
// now confirm the server has 2 todo items
cy.request('/todos')
.its('body')
.should('have.length', 2)
.and((items) => {
// confirm the returned items
})
})
A good strategy for writing targeted API tests is to use them to reach the hard-to-test code not covered by other tests. You can find such places in the code using the code coverage as a guide. Watch the
"How-to" Cypress Questions​
How do I get an element's text contents?​
Cypress commands yield jQuery objects, so you can call methods on them.
If you're trying to assert on an element's text content:
cy.get('div').should('have.text', 'foobarbaz')
If the text contains a
non-breaking space entity
then use the Unicode character \u00a0
instead of
.
<div>Hello world</div>
cy.get('div').should('have.text', 'Hello\u00a0world')
You can also use the cy.contains command which handles the non-breaking space entities
cy.contains('div', 'Hello world')
If you'd like to work with the text prior to an assertion:
cy.get('div').should(($div) => {
const text = $div.text()
expect(text).to.match(/foo/)
expect(text).to.include('foo')
expect(text).not.to.include('bar')
})
If you need to convert text to a number before checking if it is greater than 10:
cy.get('div').invoke('text').then(parseFloat).should('be.gt', 10)
If you need to hold a reference or compare values of text:
cy.get('div')
.invoke('text')
.then((text1) => {
// do more work here
// click the button which changes the div's text
cy.get('button').click()
// grab the div again and compare its previous text
// to the current text
cy.get('div')
.invoke('text')
.should((text2) => {
expect(text1).not.to.eq(text2)
})
})
jQuery's .text()
method automatically calls elem.textContent
under the hood.
If you'd like to instead use innerText
you can do the following:
cy.get('div').should(($div) => {
// access the native DOM element
expect($div.get(0).innerText).to.eq('foobarbaz')
})
This is the equivalent of Selenium's getText()
method, which returns the
innerText of a visible element.
How do I get an input's value?​
Cypress yields you jQuery objects, so you can call methods on them.
If you're trying to assert on an input's value:
// make an assertion on the value
cy.get('input').should('have.value', 'abc')
If you'd like to massage or work with the text prior to an assertion:
cy.get('input').should(($input) => {
const val = $input.val()
expect(val).to.match(/foo/)
expect(val).to.include('foo')
expect(val).not.to.include('bar')
})
If you need to hold a reference or compare values of text:
cy.get('input')
.invoke('val')
.then((val1) => {
// do more work here
// click the button which changes the input's value
cy.get('button').click()
// grab the input again and compare its previous value
// to the current value
cy.get('input')
.invoke('val')
.should((val2) => {
expect(val1).not.to.eq(val2)
})
})
How do I compare the value or state of one thing to another?​
Our Variables and Aliases guide gives you examples of doing exactly that.
Can I store an attribute's value in a constant or a variable for later use?​
Yes, and there are a couple of ways to do this. One way to hold a value or
reference is with
closures. Commonly,
users believe they have a need to store a value in a const
, var
, or let
.
Cypress recommends doing this only when dealing with mutable objects (that
change state).
For examples how to do this, please read our Variables and Aliases guide.
How do I get the native DOM reference of an element found using Cypress?​
Cypress wraps elements in jQuery so you'd get the native element from there within a .then() command.
cy.get('button').then(($el) => {
$el.get(0)
})
How do I do something different if an element doesn't exist?​
What you're asking about is conditional testing and control flow.
Please read our extensive Conditional Testing Guide which explains this in detail.
How can I make Cypress wait until something is visible in the DOM?​
DOM based commands will automatically retry and wait for their corresponding elements to exist before failing.
Cypress offers you many robust ways to query the DOM, all wrapped with retry-and-timeout logic.
Other ways to wait for an element's presence in the DOM is through timeouts
.
Cypress commands have a default timeout of 4 seconds, however, most Cypress
commands have
customizable timeout options.
Timeouts can be configured globally or on a per-command basis.
In some cases,
your DOM element will not be actionable. Cypress gives you a powerful
{force:true}
option
you can pass to most action commands.
Please read our Core Concepts Introduction to Cypress. This is the single most important guide for understanding how to test with Cypress.
How do I wait for my application to load? End-to-End Only​
We have seen many different iterations of this question. The answers can be varied depending on how your application behaves and the circumstances under which you are testing it. Here are a few of the most common versions of this question.
How do I know if my page is done loading?
When you load your application using cy.visit()
, Cypress will wait for the
load
event to fire. The cy.visit() command loads
a remote page and does not resolve until all of the external resources complete
their loading phase. Because we expect your applications to observe differing
load times, this command's default timeout is set to 60000ms. If you visit an
invalid url or a
second unique domain,
Cypress will log a verbose yet friendly error message.
In CI, how do I make sure my server has started?
You can try these modules for this use case:
How can I wait for my requests to be complete?
The prescribed way to do this is to define your routes using cy.intercept(), create aliases for these routes prior to the visit, and then you can explicitly tell Cypress which routes you want to wait on using cy.wait(). There is no magical way to wait for all of your XHRs or Ajax requests. Because of the asynchronous nature of these requests, Cypress cannot intuitively know to wait for them. You must define these routes and be able to unambiguously tell Cypress which requests you want to wait on.
Can I throttle network speeds using Cypress?​
You can throttle your network connection by accessing your Developer Tools Network panel. Additionally, you can add your own custom presets by selecting Custom > Add from the Network Conditions drawer.
We do not currently offer any options to simulate this during cypress run
.
Can I use ES7 async / await syntax?​
No. The Command API is not designed in a way that makes this possible currently. To understand how Cypress commands work, please read:
- Our Introduction to Cypress guide which explains how the Commands are designed
- Our Variables and Aliases guide which talks about patterns dealing with async code
How do I select or query for elements if my application uses dynamic classes or dynamic IDs?​
Read more about the best practices for selecting elements here.
I want to run tests only within one specific folder. How do I do this?​
You can specify which test files to run during
cypress run by
passing a glob to the --spec
flag
matching the files you want to run. You should be able to pass a glob matching
the specific folder where the tests are you want to run.
This feature is only available when using cypress run.
Is there a suggested way or best practice for how I should target elements or write element selectors?​
Yes. Read more about the best practices for selecting elements here.
Can I prevent Cypress from failing my test when my application throws an uncaught exception error?​
Yes. By default Cypress will automatically fail tests whenever an uncaught exception bubbles up out of your app.
Cypress exposes an event for this (amongst many others) that you can listen for to either:
- Debug the error instance itself
- Prevent Cypress from failing the test
This is documented in detail on the Catalog Of Events page and the recipe Handling errors.
Will Cypress fail the test when an application has unhandled rejected promise?​
By default no, Cypress does not listen to the unhandled promise rejection event in your application, and thus does not fail the test. You can set up your own listener though and fail the test, see our recipe Handling errors:
// register listener during cy.visit
it('fails on unhandled rejection', () => {
cy.visit('/', {
onBeforeLoad(win) {
win.addEventListener('unhandledrejection', (event) => {
const msg = `UNHANDLED PROMISE REJECTION: ${event.reason}`
// fail the test
throw new Error(msg)
})
},
})
})
// ALTERNATIVE: register listener for this test
it('fails on unhandled rejection', () => {
cy.on('window:before:load', (win) => {
win.addEventListener('unhandledrejection', (event) => {
const msg = `UNHANDLED PROMISE REJECTION: ${event.reason}`
// fail the test
throw new Error(msg)
})
})
cy.visit('/')
})
// ALTERNATIVE: register listener in every test
before(() => {
Cypress.on('window:before:load', (win) => {
win.addEventListener('unhandledrejection', (event) => {
const msg = `UNHANDLED PROMISE REJECTION: ${event.reason}`
// fail the test
throw new Error(msg)
})
})
})
it('fails on unhandled rejection', () => {
cy.visit('/')
})
Can I override environment variables or create configuration for different environments?​
Yes, you can pass configuration to Cypress via environment variables, CLI arguments, JSON files and other means.
Read the Environment Variables guide.
Can I override or change the default user agent the browser uses?​
Yes.
- Cypress recommends setting the
userAgent
as a configuration value in your configuration file. - It is possible to fake the
userAgent
for a singlecy.visit()
orcy.request()
by setting theuser-agent
header in the options, but this will not propagate theuserAgent
in the browser and can lead to application rendering unexpectedly.
Can I block traffic going to specific domains? I want to block Google Analytics or other providers.​
Yes.
You can set this with blockHosts
in the Cypress configuration.
Also, check out our Stubbing Google Analytics Recipe.
How can I verify that calls to analytics like Google Analytics are being made correct?​
You can stub their functions and then ensure they're being called.
Check out our Stubbing Google Analytics Recipe.
I'm trying to test a chat application. Can I run more than one browser at a time with Cypress?​
We've answered this question in detail here.
How can I modify or pass arguments used to launch the browser?​
You use the before:browser:launch
plugin
event.
Can I make cy.request() poll until a condition is met?​
Yes. You do it the same way as any other recursive loop.
Can I use the Page Object pattern?​
Yes.
If you're looking to abstract behavior or roll up a series of actions you can create reusable Custom Commands with our API. You can also use JavaScript functions to achieve this.
For those wanting to use page objects, we've highlighted the best practices for replicating the page object pattern.
Can I run a single test or group of tests?​
You can run a group of tests or a single test by placing an
.only
on a test suite or specific test.
You can run a single test file or group of tests by passing the --spec
flag to
cypress run.
How do I test uploading a file?​
It is possible to upload files in your application but it's different based on
how you've written your own upload code. The various options are detailed in the
.selectFile()
command, but in many cases the
simplest option will work:
cy.get('[data-cy="file-input"]').selectFile('cypress/fixtures/data.json')
How do I check that an email was sent out?​
Don't try to use your UI to check email. Instead opt to programmatically use 3rd party APIs or talk directly to your server. Read about this best practice here.
- If your application is running locally and is sending the emails directly through an SMTP server, you can use a temporary local test SMTP server running inside Cypress. Read the blog post "Testing HTML Emails using Cypress" for details.
- If your application is using a 3rd party email service, or you cannot stub the SMTP requests, you can use a test email inbox with an API access. Read the blog post "Full Testing of HTML Emails using SendGrid and Ethereal Accounts" for details.
Cypress can even load the received HTML email in its browser to verify the email's functionality and visual style:
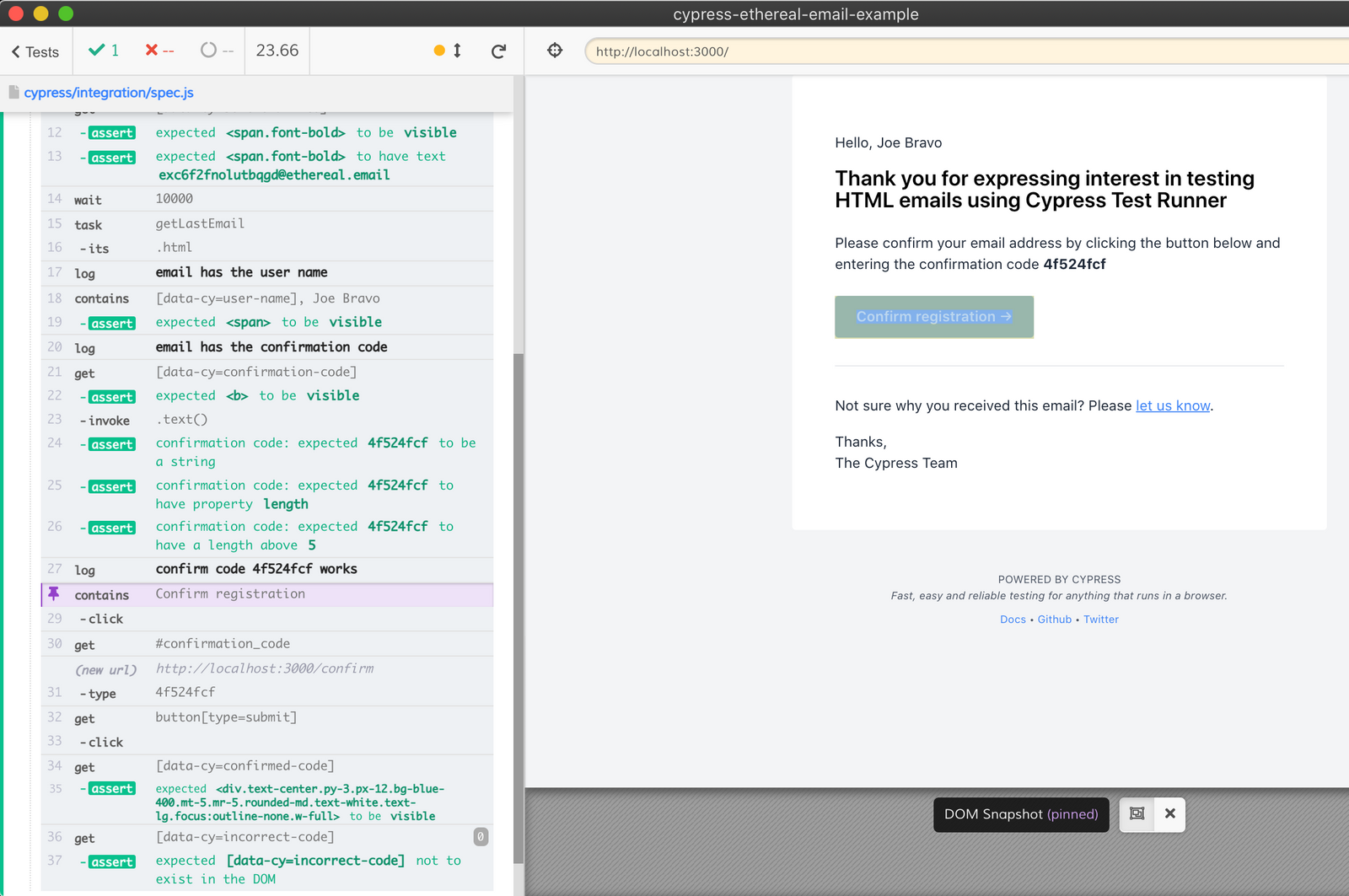
- You can use a 3rd party email service that provides temporary email addresses for testing. Some of these services even offer a Cypress plugin to access emails.
How do I wait for multiple requests to the same url?​
You should set up an alias (using .as()
) to a single
cy.intercept()
that matches all of the XHRs. You
can then cy.wait()
on it multiple times. Cypress keeps
track of how many matching requests there are.
cy.intercept('/users*').as('getUsers')
cy.wait('@getUsers') // Wait for first GET to /users/
cy.get('#list>li').should('have.length', 10)
cy.get('#load-more-btn').click()
cy.wait('@getUsers') // Wait for second GET to /users/
cy.get('#list>li').should('have.length', 20)
How do I seed / reset my database?​
You can use cy.request()
,
cy.exec()
, or cy.task()
to talk
to your back end to seed data.
You could also stub requests directly using
cy.intercept()
which avoids ever even needing to
fuss with your database.
How do I test elements inside an iframe?​
We have an open proposal to expand the APIs to support "switching into" an iframe and then back out of them.
How do I preserve cookies / localStorage in between my tests?​
By default, Cypress automatically clears all cookies, local storage and session before each test to prevent state from leaking between tests.
You can preserve session details across tests using the
cy.session()
command.
Alternatively, you can disable
testIsolation
at the suite or e2e
configuration-level to prevent the clearing of browser state.
Some of my elements animate in; how do I work around that?​
Oftentimes you can usually account for animation by asserting
.should('be.visible')
or
another assertion on
one of the elements you expect to be animated in.
// assuming a click event causes the animation
cy.get('.element').click().should('not.have.class', 'animating')
If the animation is especially long, you could extend the time Cypress waits for
the assertion to pass by increasing the timeout
of the previous command before
the assertion.
cy.get('button', { timeout: 10000 }) // wait up to 10 seconds for this 'button' to exist
.should('be.visible') // and to be visible
cy.get('.element')
.click({ timeout: 10000 })
.should('not.have.class', 'animating')
// wait up to 10 seconds for the .element to not have 'animating' class
However, most of the time you don't even have to worry about animations. Why
not? Cypress will
automatically wait for
elements to stop animating prior to interacting with them via action commands
like .click()
or .type()
.
Can I test anchor links that open in a new tab?​
Cypress does not have native multi-tab support, but new tabs can be tested with the @cypress/puppeteer plugin.
If you would like to test anchor links natively, there are lots of workarounds that enable you to test them in your application.
Read through the recipe on tab handling and links to see how to test anchor links.
Can I dynamically test multiple viewports?​
Yes, you can. We provide an example here.
Can I run the same tests on multiple subdomains? End-to-End Only​
Yes. In this example, we loop through an array of urls and make assertions on the logo.
const urls = ['https://docs.cypress.io', 'https://www.cypress.io']
describe('Logo', () => {
urls.forEach((url) => {
it(`Should display logo on ${url}`, () => {
cy.visit(url)
cy.get('#logo img').should('have.attr', 'src').and('include', 'logo')
})
})
})
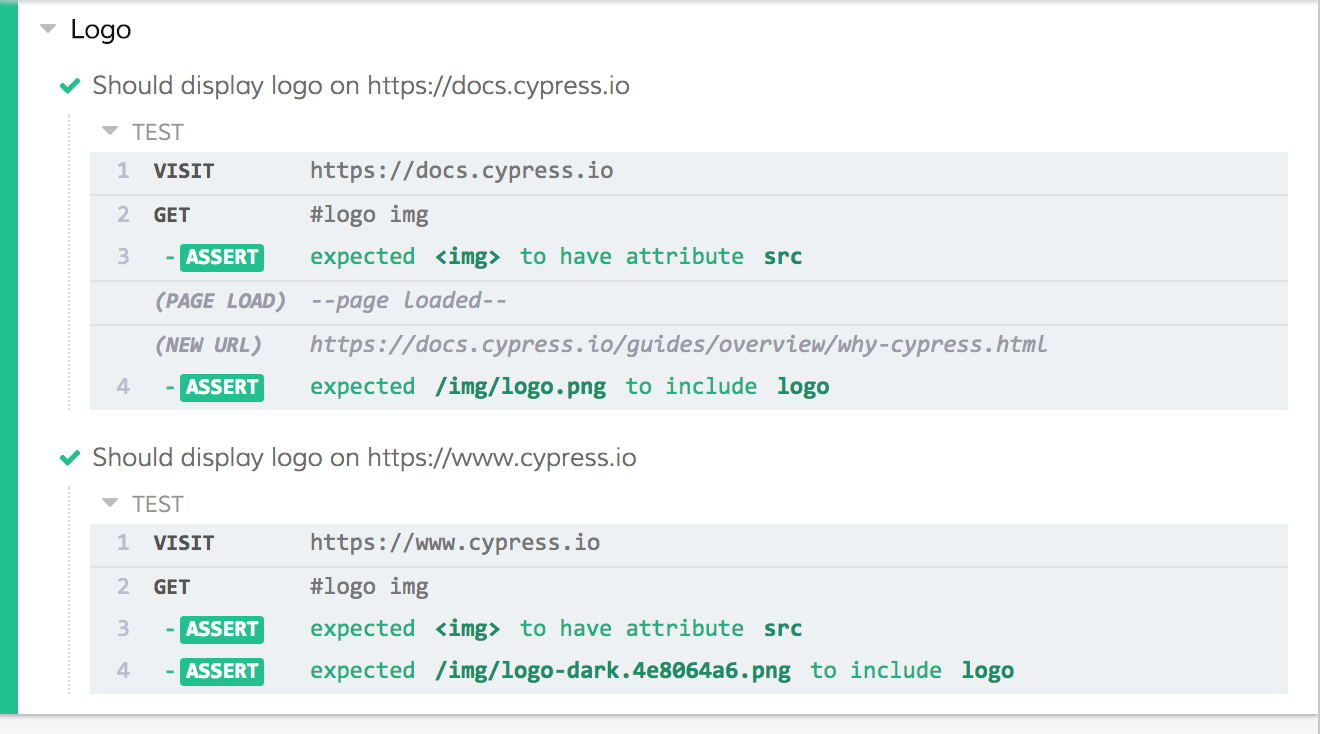
How do I require or import node modules in Cypress?​
The code you write in Cypress is executed in the browser, so you can import or require JS modules, but only those that work in a browser.
You can require
or import
them as you're accustomed to. We preprocess your
spec files with webpack and Babel.
We recommend utilizing one of the following to execute code outside of the browser.
cy.task()
to run code in Node via the setupNodeEvents functioncy.exec()
to execute a shell command
Check out the "Node Modules" example recipe.
Is there a way to give a proper SSL certificate to your proxy so the page doesn't show up as "not secure"?​
No, Cypress modifies network traffic in real time and therefore must sit between your server and the browser. There is no other way for us to achieve that.
Is there any way to detect if my app is running under Cypress?​
You can check for the existence of window.Cypress
, in your application
code.
Here's an example:
if (window.Cypress) {
// we are running in Cypress
// so do something different here
window.env = 'test'
} else {
// we are running in a regular ol' browser
}
If you want to detect if your Node.js code is running within Cypress, Cypress
sets an OS level environment variable of CYPRESS=true
. You could detect that
you're running in Cypress by looking for process.env.CYPRESS
.
Is there a way to test that a file got downloaded? I want to test that a button click triggers a download.​
There are a lot of ways to test this, so it depends. You'll need to be aware of what actually causes the download, then think of a way to test that mechanism.
If your server sends specific disposition headers which cause a browser to prompt for download, you can figure out what URL this request is made to, and use cy.request() to hit that directly. Then you can test that the server send the right response headers.
If it's an anchor that initiates the download, you could test that it has the
right href
property. As long as you can verify that clicking the button is
going to make the right HTTP request, that might be enough to test for.
Finally, if you want to really download the file and verify its contents, see our File download recipe.
In the end, it's up to you to know your implementation and to test enough to cover everything.
Is it possible to catch the promise chain in Cypress?​
No. You cannot add a .catch
error handler to a failed command.
Read more about how the Cypress commands are not Promises
Is there a way to modify the screenshots/video resolution?​
There is an open issue for more easily configuring this.
You can modify the screenshot and video size when running headlessly with this workaround.
Does Cypress support ES7?​
Yes. You can customize how specs are processed by using one of our preprocessor plugins or by writing your own custom preprocessor.
Typically you'd reuse your existing Babel and webpack configurations.
How does one determine what the latest version of Cypress is?​
There are a few ways.
- The easiest way is probably to check our changelog.
- You can also check the latest version here.
- It's also always in our repo.
When I visit my site directly, the certificate is verified, however the browser launched through Cypress is showing it as "Not Secure". Why?​
When using Cypress to test an HTTPS site, you might see a browser warning next to the browser URL. This is normal. Cypress modifies the traffic between your server and the browser. The browser notices this and displays a certificate warning. However, this is purely cosmetic and does not alter the way your application under test runs in any way, so you can safely ignore this warning. The network traffic between Cypress and the backend server still happens via HTTPS.
See also the Cross Origin Testing guide.
How do I run the server and tests together and then shutdown the server?​
To start the server, run the tests and then shutdown the server we recommend these npm tools.
I found a bug! What do I do?​
- Search existing open issues, it may already be reported!
- Update Cypress. Your issue may have already been fixed.
- Open an issue. Your best chance of getting a bug looked at quickly is to provide a repository with a reproducible bug that can be cloned and run.
What is the right balance between custom commands and utility functions?​
There is already a great section in Custom Commands guide that talks about trade-offs between custom commands and utility functions. We feel reusable functions in general are a way to go. Plus they do not confuse IntelliSense like custom commands do.
Can my tests interact with Redux / Vuex data store?​
Usually your end-to-end tests interact with the application through public
browser APIs: DOM, network, storage, etc. But sometimes you might want to make
assertions against the data held inside the application's data store. Cypress
helps you do this. Tests run right in the same browser instance and can reach
into the application's context using cy.window
. By
conditionally exposing the application reference and data store from the
application's code, you can allow the tests to make assertions about the data
store, and even drive the application via Redux actions.
- see Testing Redux Store blog post and Redux Testing recipe.
- see Vue + Vuex + REST Testing recipe.
For component testing, you have a bit more control on how you set up your providers and plugins for state stores. See the Mount API Guide for various examples on using stores with component testing.
How do I spy on console.log?​
To spy on console.log
you should use cy.stub().
- End-to-End Test
- Component Test
cy.visit('/', {
onBeforeLoad(win) {
// Stub your functions here
cy.stub(win.console, 'log').as('consoleLog')
},
})
// Other test code
cy.get('@consoleLog').should('be.calledWith', 'Hello World!')
// Stub your functions here
cy.stub(window, 'prompt').returns('my custom message')
// After that, mount your component
cy.mount(<MyComponent />)
// Other test code
cy.get('@consoleLog').should('be.calledWith', 'Hello World!')
Also, check out our
Stubbing console
Recipe.
How do I use special characters with cy.get()
?​
Special characters like /
, .
are valid characters for ids
according to the CSS spec.
To test elements with those characters in ids, they need to be escaped with
CSS.escape
or
Cypress.$.escapeSelector
.
<!doctype html>
<html lang="en">
<body>
<div id="Configuration/Setup/TextField.id">Hello World</div>
</body>
</html>
it('test', () => {
cy.visit('index.html')
cy.get(`#${CSS.escape('Configuration/Setup/TextField.id')}`).contains(
'Hello World'
)
cy.get(
`#${Cypress.$.escapeSelector('Configuration/Setup/TextField.id')}`
).contains('Hello World')
})
Note that cy.$$.escapeSelector()
doesn't work. cy.$$
doesn't refer to
jQuery
. It only queries DOM. Learn more about why
Why doesn't the instanceof Event
work?​
It might be because of the 2 different windows in the Cypress App. For more information, please check the note here.
How do I prevent application redirecting to another URL?​
Sometimes, your application might redirect the browser to another domain, losing
the Cypress's control. If the application is using window.location.replace
method to set a relative URL, try using the experimentalSourceRewriting
option described in our Experiments page.
Continuous Integration (CI/CD)​
Why do my Cypress tests pass locally but not in CI?​
There are many reasons why tests may fail in CI but pass locally. Some of these include:
- There is a problem isolated to the Electron browser (
cypress run
by default runs in the Electron browser) - A test failure in CI could be highlighting a bug in your CI build process
- Variability in timing when running your application in CI (For example, network requests that resolve within the timeout locally may take longer in CI)
- Machine differences in CI versus your local machine -- CPU resources, environment variables, etc.
To troubleshoot why tests are failing in CI but passing locally, you can try these strategies:
- Test locally with Electron to identify if the issue is specific to the browser.
- You can also identify browser-specific issues by running in a different
browser in CI with the
--browser
flag. - Review your CI build process to ensure nothing is changing with your application that would result in failing tests.
- Remove time-sensitive variability in your tests. For example, ensure a network request has finished before looking for the DOM element that relies on the data from that network request. You can leverage aliasing for this.
- Ensure video recording and/or screenshots are enabled for the CI run and compare the recording to the Command Log when running the test locally.
Why are my video recordings freezing or dropping frames when running in CI?​
Videos recorded on Continuous Integration may have frozen or dropped frames if there are not enough resources available when running the tests in your CI container. Like with any application, there needs to be the required CPU to run Cypress and record video. You can run your tests with memory and CPU logs enabled to identify and evaluate the resource utilization within your CI.
If you are experiencing this issue, we recommend switching to a more powerful CI container or provider.
What can I do if my tests crash or hang on CI?​
As some users have noted, a longer test has a higher chance of hanging or even crashing when running on CI. When a test runs for a long period of time, its commands and the application itself might allocate more memory than available, causing the crash. The exact risk of crashing depends on the application and the available hardware resources. While there is no single time limit that would solve this problem, in general we recommend splitting spec files to run in under one minute each.
You can further split individual long-running tests. For example, you can verify parts of the longer user feature in the separate tests as described in Split a very long Cypress test into shorter ones using App Actions.
How can I parallelize my runs?​
You can read more about parallelization here.
I tried to install Cypress in my CI, but I get the error: EACCES: permission denied
.​
First, make sure you have Node installed on your system.
npm
is a Node package that is installed globally by default when you install
Node and is required to install our
cypress
npm package.
Next, you'd want to check that you have the proper permissions for installing on
your system or you may need to run sudo npm install cypress
.
Is there an option to run Cypress in CI with Developer Tools open? We want to track network and console issues.​
No. There is not currently a way to run Cypress in cypress run
with Developer
Tools open. You can use Cypress Test Replay to see browser requests and console logs of tests that ran in CI.
Distinct Cypress Use Cases​
Can I use Cypress to test charts and graphs?​
Yes. You can leverage visual testing tools to test that charts and graphs are rendering as expected. For more information, check out the Visual Testing guide.
Can I test a chrome extension? How do I load my chrome extension?​
Yes. You can test your extensions by loading them when we launch the browser.
Can I test my Electron app?​
Testing your Electron app will not 'just work', as Cypress is designed to test anything that runs in a browser and Electron is a browser + Node.
That being said, we use Cypress to test our own Desktop app's front end - by stubbing events from Electron. These tests are open source so you can check them out here.
Can I test the HTML <head>
element?​
Yes, you can. While executing tests, you can view the entire
window.document
object in your open console using
cy.document(). You can even make assertions on the
<head>
element. Check out this example.
<!doctype html>
<html>
<head>
<meta charset="utf-8" />
<meta http-equiv="Content-Security-Policy" content="default-src 'self'" />
<meta name="description" content="This description is so meta" />
<title>Test the HEAD content</title>
<meta name="viewport" content="width=device-width, initial-scale=1" />
</head>
<body></body>
</html>
describe('The Document Metadata', () => {
beforeEach(() => {
cy.visit('/')
})
it('looks inside the head content using `cy.document()`', () => {
// this will yield the entire window.document object
// if you click on DOCUMENT from the command log,
// it will output the entire #document to the console
cy.document()
})
// or make assertions on any of the metadata in the head element
it('looks inside <title> tag', () => {
cy.get('head title').should('contain', 'Test the HEAD content')
})
it('looks inside <meta> tag for description', () => {
cy.get('head meta[name="description"]').should(
'have.attr',
'content',
'This description is so meta'
)
})
})
Can I check that a form's HTML form validation is shown when an input is invalid?​
You certainly can.
Test default validation error
<form>
<input type="text" id="name" name="name" required />
<button type="submit">Submit</button>
</form>
cy.get('[type="submit"]').click()
cy.get('input:invalid').should('have.length', 1)
cy.get('#name').then(($input) => {
expect($input[0].validationMessage).to.eq('Please fill out this field.')
})
Test custom validation error
<body>
<form>
<input type="email" id="email" name="email" />
<button type="submit">Submit</button>
</form>
<script>
const email = document.getElementById('email')
email.addEventListener('input', function (event) {
if (email.validity.typeMismatch) {
email.setCustomValidity('I expect an email!')
} else {
email.setCustomValidity('')
}
})
</script>
</body>
cy.get('input:invalid').should('have.length', 0)
cy.get('[type="email"]').type('not_an_email')
cy.get('[type="submit"]').click()
cy.get('input:invalid').should('have.length', 1)
cy.get('[type="email"]').then(($input) => {
expect($input[0].validationMessage).to.eq('I expect an email!')
})
Can Cypress be used for model-based testing?​
Real World App (RWA) is implemented using XState model state library.
Can Cypress be used for performance testing?​
Cypress is not built for performance testing. Because Cypress instruments the
page under test, proxies the network requests, and tightly controls the test
steps, Cypress adds its own overhead. Thus, the performance numbers you get from
Cypress tests are slower than "normal" use. Still, you can access the native
window.performance
object and grab the page time measurements, see the
Evaluate performance metrics
recipe.
Integrations with Other Tools/Frameworks/Libraries​
Can I test React applications using Cypress?​
For end-to-end testing, yes, absolutely. A good example of a fully tested React application is our Cypress RealWorld App. You can even use React DevTools while testing your application, read The easiest way to connect Cypress and React DevTools. If you really need to select React components by their name, props, or state, check out cypress-react-selector.
For component testing, we support various different frameworks like Create React App, Vite, and Next.js for React applications. See the Framework Configuration Guide for more info.
Can I use Jest snapshots?​
While there is no built-in snapshot
command in Cypress, you can make your own
snapshot assertion command. Read how to do so in our blog post
End-to-End Snapshot Testing.
We recommend using the 3rd-party module
cypress-plugin-snapshots.
For other snapshot plugins, search the Plugins page.
Can I use Testing Library?​
Absolutely! Feel free to add the
@testing-library/cypress
to your setup and use its methods like findByRole
, findByLabelText
,
findByText
, findByTestId
, and others to find the DOM elements.
The following example comes from the Testing Library's documentation
cy.findByRole('button', { name: /Jackie Chan/i }).click()
cy.findByRole('button', { name: /Button Text/i }).should('exist')
cy.findByRole('button', { name: /Non-existing Button Text/i }).should(
'not.exist'
)
cy.findByLabelText(/Label text/i, { timeout: 7000 }).should('exist')
// findAllByText _inside_ a form element
cy.get('form')
.findByText('button', { name: /Button Text/i })
.should('exist')
cy.findByRole('dialog').within(() => {
cy.findByRole('button', { name: /confirm/i })
})
Can I use Cucumber to write tests?​
Yes, you can.
While Cypress does not provide official support for Cucumber, you can use Cucumber through a community plugin. Using the plugin adds additional complexity to your testing workflow, so ensure your team understands the benefits and drawbacks before adopting Cucumber.
- read Cypress Super-patterns: How to elevate the quality of your test suite for best practices when writing Cucumber tests.
- To get started, read How to use Cypress with Cucumber BDD for step-by-step instructions for v9 and v10, or for instructions in v10 with typescript read Cucumber in Cypress: A step by step guide.
If your team is looking to do behavior-driven development using BDD's given/when/then syntax directly in Cypress instead of using Cucumber's scenarios, you might be interested in these articles:
- read Using the keywords Given/When/Then with Cypress but without Cucumber and BDD without Cucumber for recommendations for writing tests without using Cucumber.
Can I check the GraphQL network calls using Cypress?​
Yes, by using the newer API command cy.intercept() as described in the Working with GraphQL
Is there an ESLint plugin for Cypress or a list of globals?​
Yes! Check out our ESLint plugin. It will set up all the globals you need for running Cypress, including browser globals and Mocha globals.
Component Testing​
What is component testing?​
Many modern front-end UI libraries encourage writing applications using small, reusable building blocks known as components. Components start small (think buttons, images, inputs, etc.) and can be composed into larger components (order forms, date pickers, menus, etc.) and even entire pages.
Component testing is about testing an individual component in isolation from the rest of the app. This allows only having to worry about the component's functionality and not how it fits into an entire page.
How does Cypress do component testing?​
Cypress will take a component and mount it into a blank canvas. When doing so, you have direct access to the component's API, making it easier to pass in props or data and put a component in a certain state. From there, you can use the same Cypress commands, selectors, and assertions to write your tests.
Cypress supports multiple frameworks and development servers for component testing.
How does Cypress component testing compare to other options?​
When Cypress mounts a component, it does so in an actual browser and not a simulated environment like jsdom. This allows you to visually see and interact with the component as you work on it. You can use the same browser-based developer tools that you are used to when building web applications, such as element inspectors, modifying CSS, and source debugging.
Cypress Component Testing is built around the same tools and APIs that end-to-end testing uses. Anyone familiar with Cypress can immediately hop in and feel productive writing component tests without a large learning curve. Component tests can also use the vast Cypress ecosystem, plugins, and services (like Cypress Cloud) already available to complement your component tests.
What is the Mount Function?​
We ship a mount
function for each UI library that is imported from the
cypress
package. It is responsible for rendering components within Cypress's
sandboxed iframe and handling any framework-specific cleanup.
// example showing importing mount command in react
import { mount } from 'cypress/react'
While you can use the mount
function in your tests, we recommend using
cy.mount()
, which is added as a
custom command in the
cypress/support/component.js file:
import { mount } from 'cypress/react'
Cypress.Commands.add('mount', mount)
This allows you to use cy.mount()
in any component test without having to
import
the framework-specific mount command, as well as customizing it to fit
your needs. See the examples guide for each framework for info on creating a
custom cy.mount()
command.
Why isn't my component rendering as it should?​
Any global styles and fonts must be imported and made available to your component, just like in the application. See our guide on Styling Components for more information on doing so.
Why doesn't my spec show in the Specs page?​
If something appears missing from the spec list, make sure the files have the
proper extension and the specPattern
is correctly defined.
How do I fix ESLint errors for things like using the global Cypress objects?​
If you experience ESLint errors in your code editor around Cypress globals,
install the
eslint-plugin-cypress
ESLint plugin.
Why isn't TypeScript recognizing the global Cypress objects or custom cypress commands (eg: cy.mount
)?​
In some instances, TypeScript might not recognize the custom cy.mount()
command in Cypress spec files not located in the cypress directory. You will
get a compiler error specifying that the type is not found in this case.
A quick way to fix this is to include the cypress directory in your tsconfig.json options like so:
"include": [
"src",
"cypress"
]
TypeScript will monitor all files in the cypress folder and pick up the typings defined in the cypress/support/component.ts file.
Alternatively, you can move your typings to an external file and include that file in your tsconfig.json file. See our TypeScript Configuration guide for more info on doing this.
How do I get TypeScript to recognize Cypress types and not Jest types?​
For frameworks that include Jest out of the box (like Create React App), you
might run into issues where the Cypress global types for TypeScript conflict
with Jest global types (describe
, test
, it
, etc..). In this case, other
Cypress globals like Cypress
and cy
might not be working properly either.
We are currently investigating better ways to handle this, but for the time being, we recommend using a triple slash references directive to instruct the TypeScript compiler to look at the Cypress global types in each of your affected spec files:
/// <reference types="cypress" />
Alternatively, Relocate Component Specs
You can also group your Cypress and Jest tests inside separate folders (not co-located with components).
You will need to add a tsconfig.json
to the folder and specify the types the
files inside that folder should use.
Don't forget to update your
specPattern
to include the new file location.