Run Cypress in GitHub Actions
What you'll learn​
- How to set up Cypress tests in GitHub Actions
- How to cache dependencies and build artifacts
- How to parallelize Cypress tests in GitHub Actions
- How to use Cypress Cloud with GitHub Actions
GitHub offers developers Actions that provide a way to automate, customize, and execute your software development workflows within your GitHub repository. Detailed documentation is available in the GitHub Action Documentation.
Cypress GitHub Action​
Workflows can be packaged and shared as GitHub Actions. GitHub maintains many, such as the checkout and Upload/Download Artifact Actions actions used below.
The official Cypress GitHub Action is maintained by Cypress and our community to help ease the setup of Cypress in a GitHub Action. The action provides dependency installation (via npm, yarn, or pnpm), built-in caching of Node dependencies, and additional configuration options for advanced workflows.
Version Number Selection​
We recommend binding to the action's latest major version by specifying v6
when using the action.
For Example:
jobs:
cypress-run:
steps:
- uses: cypress-io/github-action@v6
Alternatively, as a mitigation strategy for unforeseen breaks, bind to a
specific
release version tag, for
example cypress-io/[email protected]
. Read the
Cypress GitHub Action documentation
for more information.
Basic Setup​
The example below is a basic CI setup and job using the
Cypress GitHub Action to
run Cypress tests within the Electron browser. This GitHub Action configuration
is placed within .github/workflows/main.yml
.
name: Cypress Tests
on: push
jobs:
cypress-run:
runs-on: ubuntu-24.04
steps:
- name: Checkout
uses: actions/checkout@v4
# Install npm dependencies, cache them correctly
# and run all Cypress tests
- name: Cypress run
uses: cypress-io/github-action@v6
with:
build: npm run build
start: npm start
How this action works:
- On push to this repository, this job will provision and start a
GitHub-hosted Ubuntu Linux instance to run the outlined
steps
for the declaredcypress-run
job within thejobs
section of the configuration. - The GitHub checkout Action is used to check out our code from our GitHub repository.
- Finally, our Cypress GitHub Action will:
- Install npm dependencies
- Build the project (
npm run build
) - Start the project web server (
npm start
) - Run the Cypress tests within our GitHub repository within Electron.
Testing on GitHub with Installed Browsers​
GitHub-hosted runners offer images
with pre-installed browsers to use for testing. The ubuntu
and windows
runners each include Google Chrome, Mozilla Firefox, and Microsoft Edge
pre-installed. The macos
runners additionally include Apple Safari. Refer to
GitHub Actions Runner Images
for current details.
Use the action's browser
parameter to select the desired browser. To change
the above example to select Chrome instead of the default browser Electron, add
browser: chrome
as follows.
- name: Cypress run
uses: cypress-io/github-action@v6
with:
build: npm run build
start: npm start
browser: chrome
For more examples, see the action's Browser section.
If you are specifying a browser in a parallel job, see Specifying Browsers in Parallel Builds for more info on how to avoid errors during runs due to GitHub runner images being updated with the latest browsers.
Testing with Cypress Docker Images​
GitHub Actions provides the option to specify a container image for the job. Cypress offers various Docker Images for running Cypress locally and in CI.
Note that GitHub Actions requires using a Linux runner when specifying a container image. It does not support running Docker images on Windows or macOS runners.
Below, we extend the previous example by adding the container
attribute using a
cypress/browsers
Docker image which also includes Google Chrome.
We are using the Node.js short-form tag to select a cypress/browsers
image
built with the corresponding Node.js version.
To specify instead an exact set of browser versions, visit the
Docker Hub cypress/browsers page and view the available long-form tags,
for example cypress/browsers:node-22.15.0-chrome-136.0.7103.92-1-ff-138.0.1-edge-136.0.3240.50-1
.
Using a Cypress Docker image allows our tests to execute without any influence from
browser version changes in the GitHub runner image.
name: Cypress Tests using Cypress Docker Image
on: push
jobs:
cypress-run:
runs-on: ubuntu-24.04
container:
image: cypress/browsers:22.15.0
options: --user 1001
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Cypress run
uses: cypress-io/github-action@v6
with:
build: npm run build
start: npm start
browser: chrome
If you are testing with Firefox, you must specify the non-root user 1001
as above. Refer to Firefox not found for more information.
Caching Dependencies and Build Artifacts​
When working with actions that have multiple jobs, it is recommended to have an initial "install" job that will download any dependencies and build your app, and then cache these assets for use later by subsequent jobs.
The Cypress GitHub Action will automatically cache and restore your Node dependencies for you.
For build assets, you will need to cache and restore them manually.
The install
job below uses the
upload-artifact
action and saves the state of the build
directory for the cypress-run
worker
job.
The
download-artifact
action retrieves the build
directory saved in the install
job, as seen below
in the cypress-run
worker job.
name: Cypress Tests with Dependency and Artifact Caching
on: push
jobs:
install:
runs-on: ubuntu-24.04
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Cypress install
uses: cypress-io/github-action@v6
with:
# Disable running of tests within install job
runTests: false
build: npm run build
- name: Save build folder
uses: actions/upload-artifact@v4
with:
name: build
if-no-files-found: error
path: build
cypress-run:
runs-on: ubuntu-24.04
needs: install
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Download the build folder
uses: actions/download-artifact@v4
with:
name: build
path: build
- name: Cypress run
uses: cypress-io/github-action@v6
with:
start: npm start
browser: chrome
View GitHub's guide on Storing workflow data as artifacts for more info.
Parallelization​
Cypress Cloud offers the ability to parallelize and group test runs along with additional insights and analytics for Cypress tests.
Using parallelization with the Cypress GitHub Action requires setting up recording to Cypress Cloud.
GitHub Actions offers a matrix strategy for declaring different job configurations for a single job definition. Jobs declared within a matrix strategy can run in parallel, which enables us to run multiples instances of Cypress at the same time, as we will see later in this section.
Before diving into an example of a parallelization setup, it is important to understand the two different types of GitHub Action jobs that we will declare:
- Install Job: A job that installs and caches dependencies that will be used by subsequent jobs later in the GitHub Action workflow.
- Worker Job: A job that handles the execution of Cypress tests and depends on the install job.
Install Job​
The separation of installation from test running is necessary when running parallel jobs. It allows for the reuse of various build steps aided by caching.
First, we'll define the install
step that will be used by the worker jobs
defined in the matrix strategy.
For the steps
, notice that we pass runTests: false
to the Cypress GitHub
Action to instruct it only to install and cache Cypress and npm dependencies
without running the tests.
The
upload-artifact
action will save the state of the build
directory for the worker jobs.
name: Cypress Tests
on: push
jobs:
install:
runs-on: ubuntu-24.04
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Cypress install
uses: cypress-io/github-action@v6
with:
# Disable running of tests within install job
runTests: false
build: npm run build
- name: Save build folder
uses: actions/upload-artifact@v4
with:
name: build
if-no-files-found: error
path: dist
Worker Jobs​
Next, we define the worker job named "cypress-run" that will run Cypress tests as part of a parallelized matrix strategy.
The download-artifact
action will retrieve the dist directory saved in the
install job.
name: Cypress Tests
on: push
jobs:
install:
# ... omitted install job from above
cypress-run:
runs-on: ubuntu-24.04
needs: install
strategy:
# don't fail the entire matrix on failure
fail-fast: false
matrix:
# run copies of the current job in parallel
containers: [1, 2, 3, 4, 5]
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Download the build folder
uses: actions/download-artifact@v4
with:
name: build
- name: Cypress run
uses: cypress-io/github-action@v6
with:
record: true
parallel: true
group: 'UI-Chrome'
start: npm start
If a Docker container was used in the install job, the same Docker container must also be used in the worker jobs.
Setting up Parallelization​
To set up multiple containers to run in parallel, the matrix option of the strategy configuration can be set to containers: [1, 2, 3, 4, 5], where the number of items defined in the containers array will be how many instances of the job will start up.
For instance, containers: [1, 2, 3, 4, 5]
will provision five worker instances
to run in parallel. For our purposes, the array's values are arbitrary and
aren't used in the steps.
Specifying Browsers in Parallel Builds​
When GitHub deploys new runner image versions containing updated browser versions, and the deployment is still in progress, the workflow "Set up job" phase randomly uses either an old or a new runner image version. Your test run might fail if Cypress Cloud detects differences in the browser versions between parallel jobs.
To work around this issue, and if you specify a browser other than the default Electron browser in parallel mode, we recommend using a cypress/browsers Docker image which then uses one consistent browser version. This shields the workflow from browser version changes due to possible incomplete GitHub runner image deployments.
As mentioned in Testing with Cypress Docker Images, this option is only available with GitHub Actions Linux runners.
Using Cypress Cloud with GitHub Actions​
In the GitHub Actions configuration, we have defined in the previous section, we are leveraging three useful features of Cypress Cloud:
-
Recording test results with the
record: true
option to Cypress Cloud:- In-depth and shareable test reports.
- Visibility into test failures via quick access to Test Replay, error messages, stack traces, screenshots, videos, and contextual details.
- Integrating testing with the pull-request (PR) process via commit status check guards and convenient test report comments.
- Detecting flaky tests and surfacing them via Slack alerts or GitHub PR status checks.
-
Parallelizing test runs and optimizing their execution via intelligent load-balancing of test specs across CI machines with the
parallel: true
option. -
Organizing and consolidating multiple
cypress run
calls by labeled groups into a single report within Cypress Cloud. In the example above, we use thegroup: "UI-Chrome"
option to organize all UI tests for the Chrome browser into a group labeled "UI - Chrome" in the Cypress Cloud report.
Cypress Real World Example with GitHub Actions​
A complete CI workflow against multiple browsers, viewports, and operating systems is available in the Cypress Real World App.
Clone the Real World App (RWA) and refer to the .github/workflows/main.yml file.
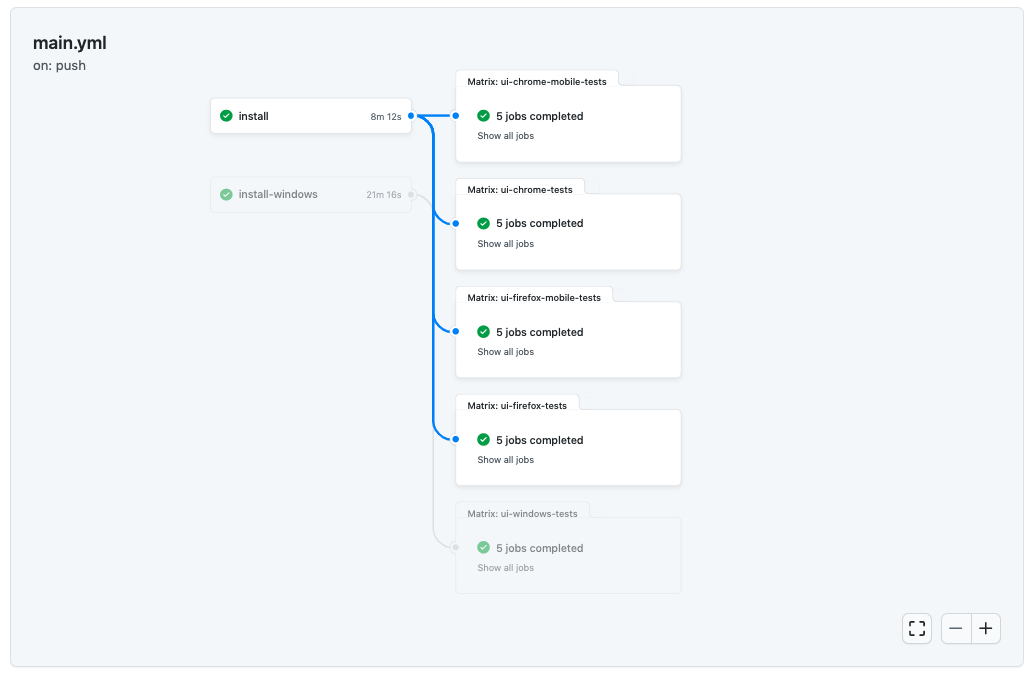
To see additional how-to examples, you can also refer to our Cypress GitHub Action repo.
Common Problems and Solutions​
Re-run jobs passing with empty tests​
We recommend passing the GITHUB_TOKEN
secret (created by the GH Action
automatically) as an environment variable. This will allow the accurate
identification of each build to avoid confusion when re-running a build.
name: Cypress tests
on: push
jobs:
cypress-run:
name: Cypress run
runs-on: ubuntu-24.04
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Cypress run
uses: cypress-io/github-action@v6
with:
record: true
env:
# pass GitHub token to detect new build vs re-run build
GITHUB_TOKEN: ${{secrets.GITHUB_TOKEN}}
Pull requests commit message is merge SHA into SHA
​
You can overwrite the commit message sent to Cypress Cloud by setting an environment variable. See Issue #124 for more details.
name: Cypress tests
on: push
jobs:
cypress-run:
name: Cypress run
runs-on: ubuntu-24.04
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Cypress run
uses: cypress-io/github-action@v6
with:
record: true
env:
# overwrite commit message sent to Cypress Cloud
COMMIT_INFO_MESSAGE: ${{github.event.pull_request.title}}
# re-enable PR comment bot
COMMIT_INFO_SHA: ${{github.event.pull_request.head.sha}}
We also recommend adding COMMIT_INFO_SHA
to re-enable
Cypress bot PR comments.
See
this comment
for more details.