Launching Browsers
What you'll learn​
- How to launch browsers in Cypress
- How to customize the list of available browsers
- How to troubleshoot browser launching issues
- Differences between Cypress browsers and regular browser environments
When you run tests in Cypress, we launch a browser for you. This enables us to:
- Create a clean, pristine testing environment.
- Access the privileged browser APIs for automation.
Cypress currently supports Firefox and Chrome-family browsers (including Edge and Electron). To run tests optimally across these browsers in CI, check out the strategies demonstrated in the cross browser Testing guide.
Browsers​
When Cypress is initially launched, you can choose to test your application using number of browsers including:
- Chrome
- Chrome Beta
- Chrome Canary
- Chrome for Testing
- Chromium
- Edge
- Edge Beta
- Edge Canary
- Edge Dev
- Electron
- Firefox
- Firefox Developer Edition
- Firefox Nightly
- WebKit (Experimental)
Cypress automatically detects available browsers on your OS. You can switch the browser by using the drop down near the top right corner:
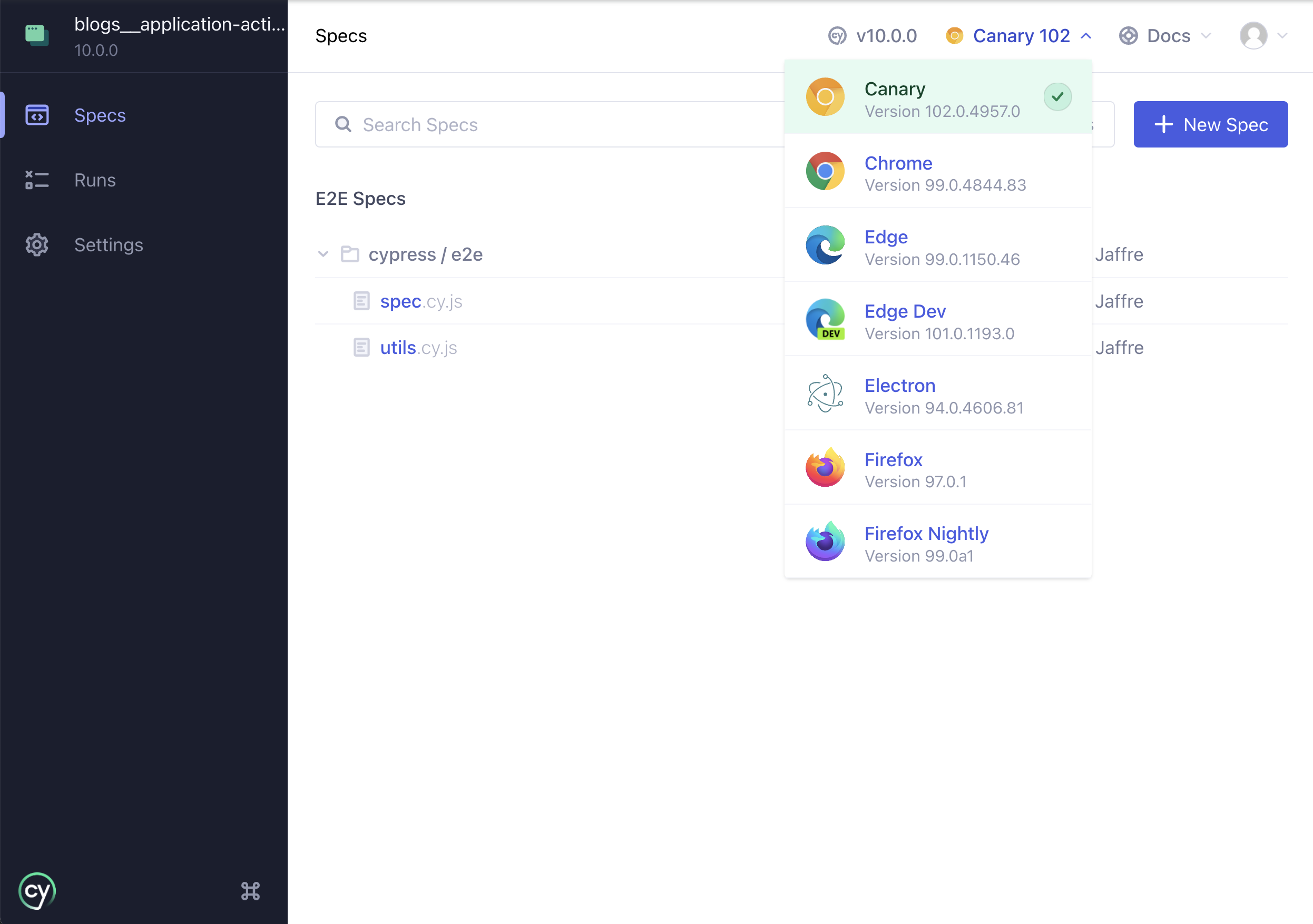
Browser versions supported​
Cypress officially supports the latest 3 major versions of Chrome, Firefox, and Edge. (For example, if the stable release of Chrome was 130, Cypress would officially support Chrome 128, 129, and 130.)
See each browser's official release schedule for more information.
Download specific Chrome version​
The Chrome browser is evergreen - meaning it will automatically update itself, sometimes causing a breaking change in your automated tests. You can use the information in Download Chromium to download a specific released version of Chrome for Testing or Chromium for every platform.
Electron Browser​
In addition to the browsers found on your system, you'll notice that Electron is an available browser. The Electron browser is a version of Chromium that comes with Electron.
The Electron browser has the advantage of coming baked into Cypress and does not need to be installed separately.
By default, when running cypress run from the CLI, we will launch all browsers headlessly.
You can also launch Electron headed:​
cypress run --headed
Because Electron is the default browser - it is typically run in CI. If you are
seeing failures in CI, to easily debug them you may want to run locally with the
--headed
option.
Chrome Browsers​
All Chrome* flavored browsers are detected and supported by Cypress.
You can launch Chrome like this:
cypress run --browser chrome
To use this command in CI, you need to install the browser you want - or use one of our docker images.
By default, we will launch Chrome headlessly during cypress run
. To run
Chrome headed, you can pass the --headed
argument to cypress run
.
You can also launch Chromium:
cypress run --browser chromium
Or Chrome Beta:
cypress run --browser chrome:beta
Or Chrome Canary:
cypress run --browser chrome:canary
Or Chrome for Testing:
cypress run --browser chrome-for-testing
Edge Browsers​
Microsoft Edge-family (Chromium-based) browsers are supported by Cypress.
You can launch Microsoft Edge like this:
cypress run --browser edge
Or Microsoft Edge Beta:
cypress run --browser edge:beta
Or Microsoft Edge Canary:
cypress run --browser edge:canary
Or Microsoft Edge Dev:
cypress run --browser edge:dev
Firefox Browsers​
Firefox-family browsers are supported by Cypress.
You can launch Firefox like this:
cypress run --browser firefox
Or Firefox Developer/Nightly Edition:
cypress run --browser firefox:dev
cypress run --browser firefox:nightly
To use this command in CI, you need to install these other browsers - or use one of our docker images.
By default, we will launch Firefox headlessly during cypress run
. To run
Firefox headed, you can pass the --headed
argument to cypress run
.
Mozilla geckodriver​
Cypress requires the Mozilla geckodriver to launch Firefox. To meet this requirement, the Cypress binary uses the separate npm wrapper package geckodriver to provide the Mozilla geckodriver. The wrapper downloads the latest driver version if it does not find any driver version cached locally.
Retrieving the driver may fail if you are operating Cypress in an air-gapped environment without Internet connectivity and you do not have a cached driver version available.
To avoid this issue, use a current
Cypress Docker image cypress/browsers
or cypress/included
, built with Firefox 139, or above.
These images include a Mozilla geckodriver version pre-installed.
Using cypress/factory:5.9.0
, or above, you can also build your own custom Cypress Docker image that includes a Mozilla geckodriver version.
Refer to the cypress/factory
documentation for instructions on building custom images.
If you need to work without Docker, refer to the npm wrapper package geckodriver documentation for information about how to define a custom path for the driver or how to refer to a local CDN mirror site. Download the Mozilla geckodriver from the releases location.
Webdriver BiDi and CDP Deprecation​
Since Firefox 129, Chrome DevTools Protocol (CDP) has been deprecated in Firefox. In Firefox 135 and above, Cypress defaults to automating the Firefox browser with WebDriver BiDi. Cypress will no longer support CDP within Firefox in the future and is planned for removal in Cypress 15.
If CDP still needs to be used, you can force enablement via the FORCE_FIREFOX_CDP=1
environment variable, regardless of Firefox version. For example:
FORCE_FIREFOX_CDP=1 npx cypress run --browser firefox
WebKit (Experimental)​
Cypress has experimental support for WebKit,
Safari's browser engine. Testing your app with WebKit is representative of how
your app would run in Safari. To opt-in to experimentalWebKitSupport
, follow
these steps:
- Add
experimentalWebKitSupport: true
to your configuration to enable the experiment. - For installation on Linux, refer to Linux Dependencies below.
- Install the
playwright-webkit
npm package in your repo to acquire WebKit itself:npm install playwright-webkit --save-dev
- Now, you should be able to use WebKit like any other browser. For example, to
record with WebKit in CI:
cypress run --browser webkit --record # ...
We built this experiment on top of the Playwright WebKit browser as a stepping stone towards creating a better UX with Cypress-provided browsers in the future. Thank you, Playwright contributors.
WebKit support is experimental, so you may encounter issues. If you encounter an issue not on the "Known Issues" list, please open an issue on the GitHub repository.
Linux Dependencies​
WebKit requires additional dependencies to run on Linux. To install the required dependencies, run this:
npx playwright install-deps webkit
Known Issues with experimentalWebKitSupport
​
cy.origin()
is not yet supported.cy.intercept()
'sforceNetworkError
option is disabled.- When using
experimentalSingleTabRunMode
with video recording in WebKit, only the video for the first spec is recorded. - Some differences in
cy.type()
behavior:textInput
events are missing thedata
propertybeforeinput
events are missing theinputType
propertycy.type('{uparrow}')
andcy.type('{downarrow}')
on aninput[type=number]
do not round to the neareststep
specified
- Stack traces may be missing some function names and location information.
- See issues labeled
experiment: webkit
for a complete list.
Launching by a path​
You can launch any supported browser by specifying a path to the binary:
cypress run --browser /usr/bin/chromium
cypress open --browser /usr/bin/chromium
Cypress will automatically detect the type of browser supplied and launch it for you.
See the Command Line guide for more information about the --browser
arguments
Having trouble launching a browser? Check out our troubleshooting guide
Customize available browsers​
Sometimes you might want to modify the list of browsers found before running tests.
For example, your web application might only be designed to work in a Chrome browser, and not inside the Electron browser.
In the setupNodeEvents function, you can
filter the list of browsers passed inside the config
object and return the
list of browsers you want available for selection during cypress open
.
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
// setupNodeEvents can be defined in either
// the e2e or component configuration
e2e: {
setupNodeEvents(on, config) {
// inside config.browsers array each object has information like
// {
// name: 'chrome',
// channel: 'canary',
// family: 'chromium',
// displayName: 'Chrome Canary',
// version: '133.0.6890.0',
// path:
// '/Applications/Google Chrome Canary.app/Contents/MacOS/Canary',
// majorVersion: 133
// }
return {
browsers: config.browsers.filter(
(b) => b.family === 'chromium' && b.name !== 'electron'
),
}
},
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
// setupNodeEvents can be defined in either
// the e2e or component configuration
e2e: {
setupNodeEvents(on, config) {
// inside config.browsers array each object has information like
// {
// name: 'chrome',
// channel: 'canary',
// family: 'chromium',
// displayName: 'Chrome Canary',
// version: '133.0.6890.0',
// path:
// '/Applications/Google Chrome Canary.app/Contents/MacOS/Canary',
// majorVersion: 133
// }
return {
browsers: config.browsers.filter(
(b) => b.family === 'chromium' && b.name !== 'electron'
),
}
},
},
})
When you open Cypress in a project that uses the above modifications to the
setupNodeEvents
function, Electron will no longer display in the list of
available browsers.
If you return an empty list of browsers or browsers: null
, the default list
will be restored automatically.
If you have installed a Chromium-based browser like Brave, Vivaldi you can add them to the list of returned browsers. Here is a configuration that inserts a local Brave browser into the returned list.
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
const execa = require('execa')
const findBrowser = () => {
// the path is hard-coded for simplicity
const browserPath =
'/Applications/Brave Browser.app/Contents/MacOS/Brave Browser'
return execa(browserPath, ['--version']).then((result) => {
// STDOUT will be like "Brave Browser 77.0.69.135"
const [, version] = /Brave Browser (\d+\.\d+\.\d+\.\d+)/.exec(result.stdout)
const majorVersion = parseInt(version.split('.')[0])
return {
name: 'Brave',
channel: 'stable',
family: 'chromium',
displayName: 'Brave',
version,
path: browserPath,
majorVersion,
}
})
}
module.exports = defineConfig({
// setupNodeEvents can be defined in either
// the e2e or component configuration
e2e: {
setupNodeEvents(on, config) {
return findBrowser().then((browser) => {
return {
browsers: config.browsers.concat(browser),
}
})
},
},
})
import { defineConfig } from 'cypress'
import execa from 'execa'
const findBrowser = () => {
// the path is hard-coded for simplicity
const browserPath =
'/Applications/Brave Browser.app/Contents/MacOS/Brave Browser'
return execa(browserPath, ['--version']).then((result) => {
// STDOUT will be like "Brave Browser 77.0.69.135"
const [, version] = /Brave Browser (\d+\.\d+\.\d+\.\d+)/.exec(result.stdout)
const majorVersion = parseInt(version.split('.')[0])
return {
name: 'Brave',
channel: 'stable',
family: 'chromium',
displayName: 'Brave',
version,
path: browserPath,
majorVersion,
}
})
}
export default defineConfig({
// setupNodeEvents can be defined in either
// the e2e or component configuration
e2e: {
setupNodeEvents(on, config) {
return findBrowser().then((browser) => {
return {
browsers: config.browsers.concat(browser),
}
})
},
},
})
Once selected, the Brave browser is detected using the same approach as any
other browser of the chromium
family.
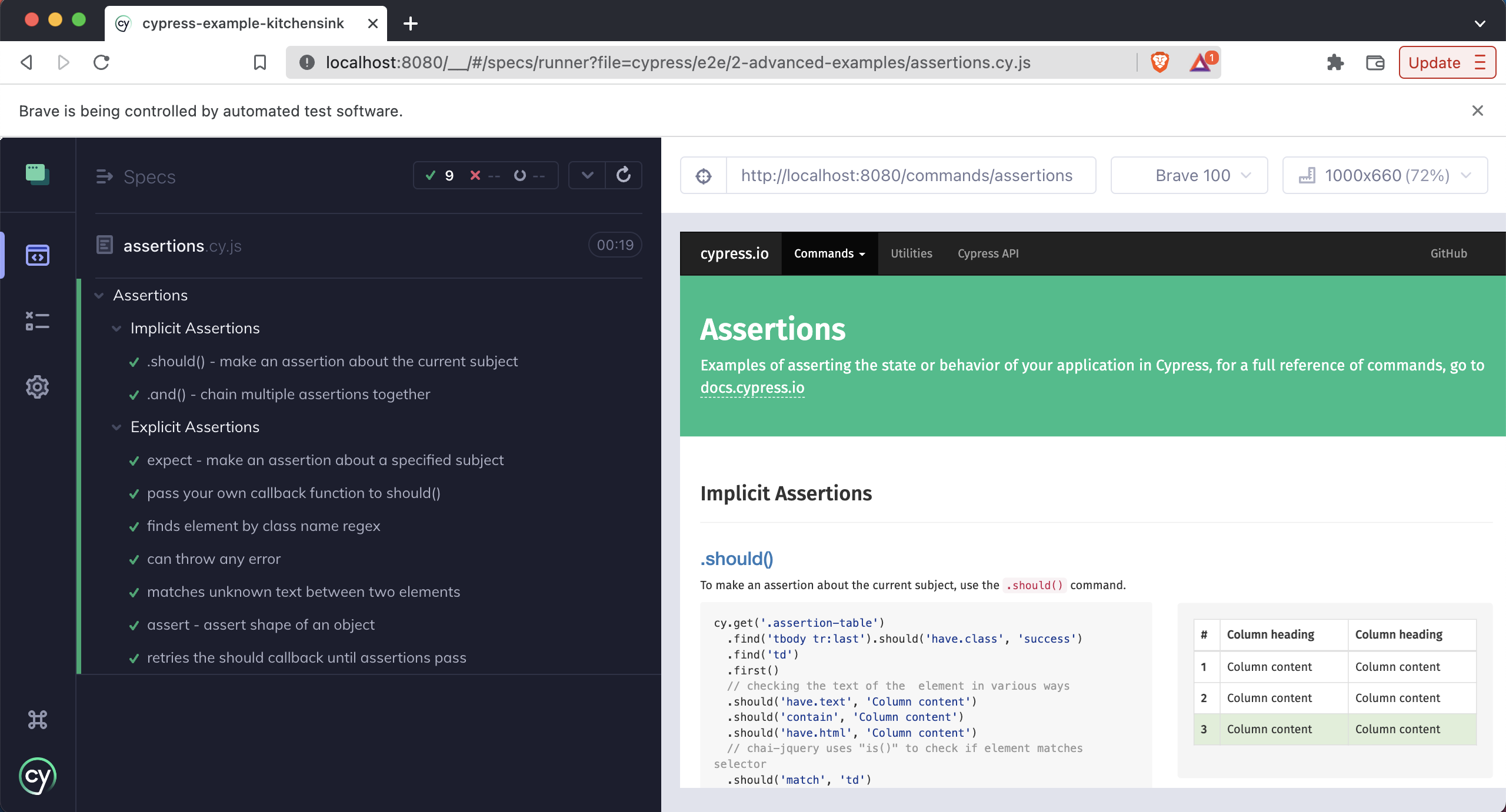
If you modify the list of browsers, you can see the resolved configuration in the Settings tab.
Unsupported Browsers​
Some browsers such as Internet Explorer are not currently supported.
Browser Environment​
Cypress launches the browser in a way that's different from a regular browser environment. But it launches in a way that we believe makes testing more reliable and accessible.
Launching Browsers​
When Cypress goes to launch your browser it will give you an opportunity to modify the arguments used to launch the browser.
This enables you to do things like:
- Load your own extension
- Enable or disable experimental features
This part of the API is documented here.
Cypress Profile​
Cypress generates its own isolated profile apart from your normal browser
profile. This means things like history
entries, cookies
, and
3rd party extensions
from your regular browsing session will not affect your
tests in Cypress.
That's no problem - you have to reinstall them once in the Cypress launched browser. We'll continue to use this Cypress testing profile on subsequent launches so all of your configuration will be preserved.
Extra Tabs​
Any extra tabs (i.e. tabs other than the one opened by Cypress) will be closed between tests. We recommend using your own browser instead of the one launched by Cypress for general-purpose browsing.
Disabled Barriers​
Cypress automatically disables certain functionality in the Cypress launched browser that tend to get in the way of automated testing.
The Cypress launched browser automatically:​
- Ignores certificate errors.
- Allows blocked pop-ups.
- Disables 'Saving passwords'.
- Disables 'Autofill forms and passwords'.
- Disables asking to become your primary browser.
- Disables device discovery notifications.
- Disables language translations.
- Disables restoring sessions.
- Disables background network traffic.
- Disables background and renderer throttling.
- Disables prompts requesting permission to use devices like cameras or mics
- Disables user gesture requirements for autoplaying videos.
You can see all of the default chrome command line switches we send here.
Browser Icon​
You might notice that if you already have the browser open you will see two of the same browser icons in your dock.
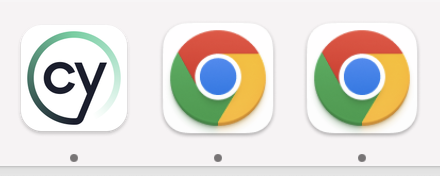
We understand that when Cypress is running in its own profile it can be difficult to tell the difference between your normal browser and Cypress.
For this reason you may find downloading and using a browser's release channel versions (Dev, Canary, etc) useful. These browsers have different icons from the standard stable browser, making them more distinguishable. You can also use the bundled Electron browser, which does not have a dock icon.
Additionally, in Chrome-based browsers, we've made the browser spawned by Cypress look different than regular sessions. You'll see a darker theme around the chrome of the browser. You'll always be able to visually distinguish these.

Troubleshooting​
Having issues launching installed browsers? Read more about troubleshooting browser launching