Vue Component Testing
What you'll learn​
- How to set up component tests in Vue
- How to configure Cypress for Vue projects
- How to use Cypress with Vue CLI, Nuxt, Vite, and custom Webpack config
Framework Support​
Cypress Component Testing supports Vue 2+ with the following frameworks:
Tutorial​
Visit the Getting Started Guide for a step-by-step tutorial on adding component testing to any project and how to write your first tests.
Installation​
To get up and running with Cypress Component Testing in Vue, install Cypress into your project:
- npm
- yarn
- pnpm
npm install cypress --save-dev
yarn add cypress --dev
pnpm add --save-dev cypress
Open Cypress:
- npm
- yarn
- pnpm
npx cypress open
yarn cypress open
pnpm cypress open
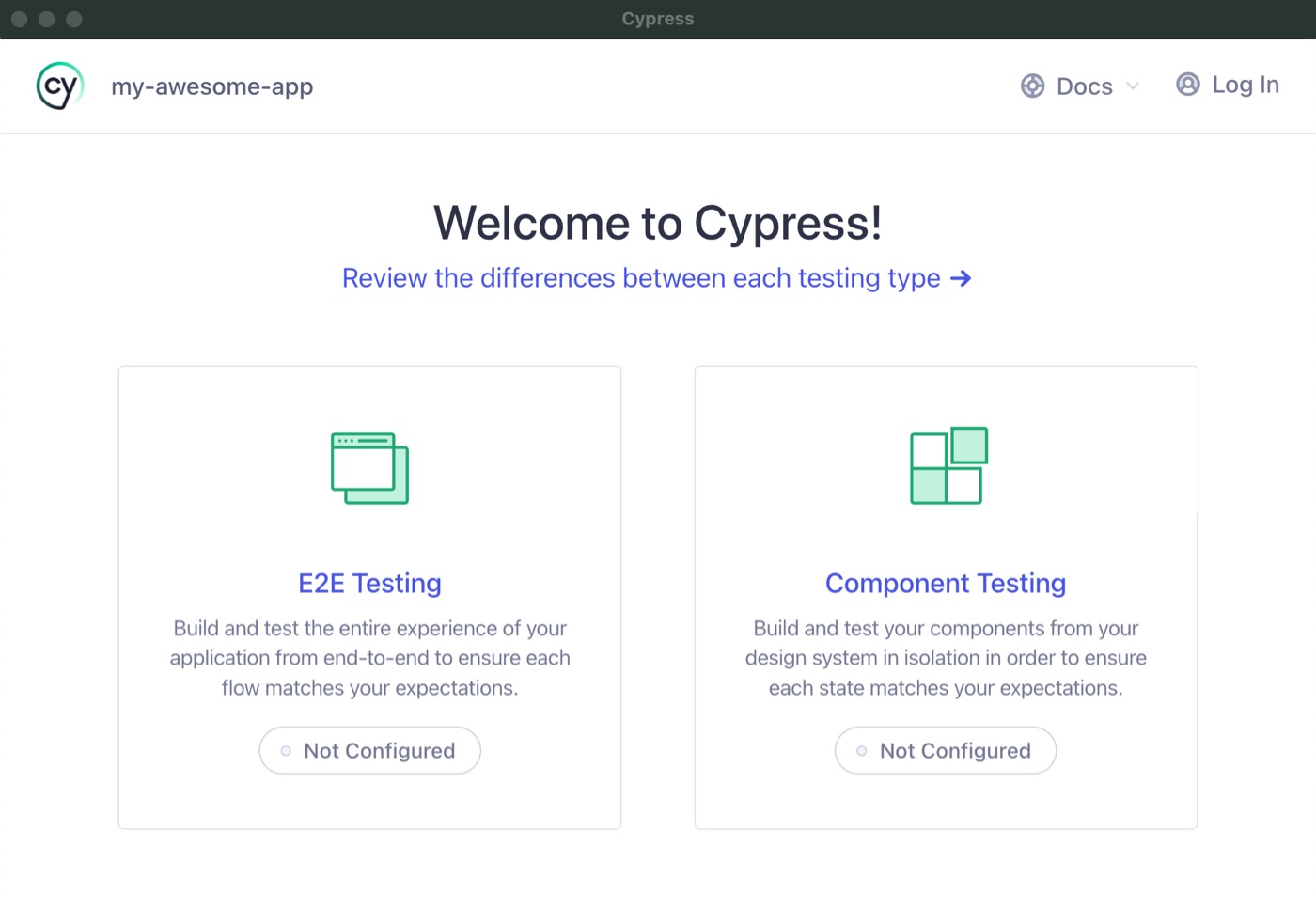
Choose Component Testing
The Cypress Launchpad will guide you through configuring your project.
For a step-by-step guide on how to create a component test, refer to the Getting Started guide.
For usage and examples, visit the Vue Examples guide.
Framework Configuration​
Cypress Component Testing works out of the box with Vue CLI, Nuxt, Vite, and a custom Webpack config. Cypress will automatically detect one of these frameworks during setup and configure them properly. The examples below are for reference purposes.
Vue CLI​
Cypress Component Testing works with Vue CLI.
Vue CLI Configuration​
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
component: {
devServer: {
framework: 'vue-cli',
bundler: 'webpack',
},
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
component: {
devServer: {
framework: 'vue-cli',
bundler: 'webpack',
},
},
})
If you use the Vue CLI's PWA feature, there is a known caveat regarding configuration. See here for more information.
Sample Vue CLI Apps​
Nuxt​
Cypress Component Testing works with Nuxt 2. Nuxt 3 is not yet supported.
Nuxt is currently in alpha support for component testing.
Nuxt Configuration​
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
component: {
devServer: {
framework: 'nuxt',
bundler: 'webpack',
},
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
component: {
devServer: {
framework: 'nuxt',
bundler: 'webpack',
},
},
})
Nuxt Sample Apps​
Vue with Vite​
Cypress Component Testing works with Vue apps that use Vite 2+ as the bundler.
Vite Configuration​
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
module.exports = defineConfig({
component: {
devServer: {
framework: 'vue',
bundler: 'vite',
},
},
})
import { defineConfig } from 'cypress'
export default defineConfig({
component: {
devServer: {
framework: 'vue',
bundler: 'vite',
},
},
})
Vue Vite Sample Apps​
Vue with Webpack​
Cypress Component Testing works with Vue apps that use Webpack 4+ as the bundler.
Webpack Configuration​
- cypress.config.js
- cypress.config.ts
const { defineConfig } = require('cypress')
const webpackConfig = require('./webpack.config')
module.exports = defineConfig({
component: {
devServer: {
framework: 'vue',
bundler: 'webpack',
// optionally pass in webpack config
webpackConfig,
webpackConfig: async () => {
// ... do things ...
const modifiedConfig = await injectCustomConfig(baseConfig)
return modifiedConfig
},
},
},
})
import { defineConfig } from 'cypress'
import webpackConfig from './webpack.config'
export default defineConfig({
component: {
devServer: {
framework: 'vue',
bundler: 'webpack',
// optionally pass in webpack config
webpackConfig,
webpackConfig: async () => {
// ... do things ...
const modifiedConfig = await injectCustomConfig(baseConfig)
return modifiedConfig
},
},
},
})
If you don't provide one, Cypress will try to infer your webpack config. If
Cypress cannot or you want to make modifications to your config, you can pass it
in manually via the webpackConfig
option.